Cropping images is an essential skill in digital media. With Python, you can easily crop images to focus on specific parts or to create thumbnails. This guide will help you learn the steps needed to crop
Setting Up Your Python Environment
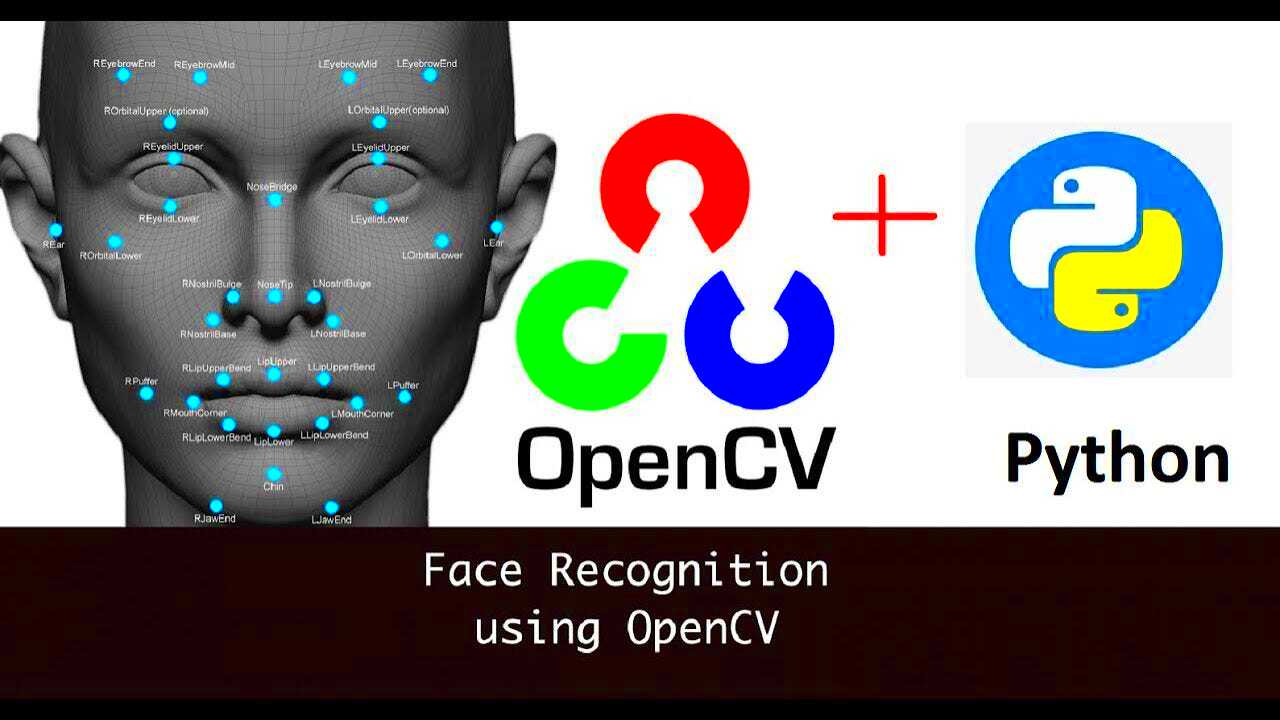
Before diving into cropping images, you need to set up your Python environment. Here are the steps to ensure you're ready:
- Install Python: Download the latest version of Python from the official website and install it on your system.
- Install Required Libraries: You will need the Pillow library, a powerful image processing library. You can install it using pip. Open your command line and type:
pip install Pillow
This command will download and install the library, allowing you to work with images easily.
Optional: You might also want to use an IDE like PyCharm or Visual Studio Code to write your code. They provide features that make coding easier, such as syntax highlighting and code suggestions.
Understanding Image Cropping Basics
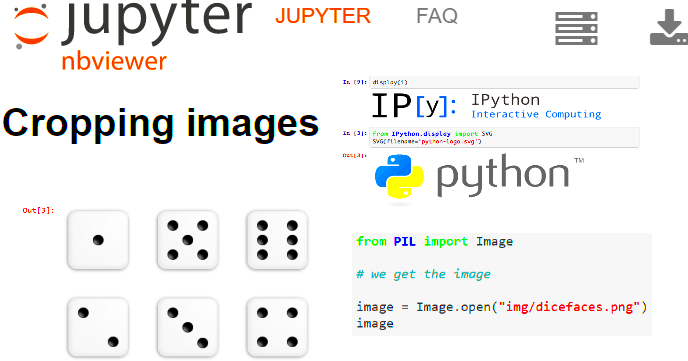
Before you start cropping images, it's essential to understand a few basic concepts:
- What is Cropping? Cropping refers to removing unwanted outer areas from an image. This helps focus on a particular subject.
- Image Format: Ensure your image is in a supported format such as JPEG, PNG, or BMP.
- Coordinate System: Images are represented as a grid of pixels. The top-left corner is (0,0), with coordinates increasing to the right and downward.
By keeping these concepts in mind, you will be better prepared to crop images effectively. Understanding the coordinate system is crucial since you'll need to specify the area you want to crop using these coordinates.
Using the PIL Library for Image Processing
The Python Imaging Library (PIL), now maintained under the name Pillow, is a fantastic tool for image processing in Python. With this library, you can easily manipulate images, including cropping, resizing, and filtering. It provides a user-friendly interface for working with various image formats. Let’s explore some key features of the PIL library:
- Opening Images: You can open images using a simple command, which makes it easy to work with different file types.
- Basic Image Operations: Apart from cropping, you can also resize, rotate, and flip images.
- Image Filters: Apply various filters to enhance your images, like blur, sharpen, or convert to grayscale.
- Saving Images: After processing your image, you can save it in different formats.
To get started with the Pillow library, make sure you have it installed (as mentioned earlier). Then, you can easily import it into your Python scripts using:
from PIL import Image
This line allows you to access all the functionality of the Pillow library. In the next sections, we'll put this knowledge to practical use by cropping images step-by-step.
Step-by-Step Cropping Process
Cropping images using Python with the Pillow library is straightforward. Here’s a step-by-step guide to help you through the process:
- Open the Image: Use the
Image.open()
function to load your image. - Define the Crop Area: Determine the area you want to crop. You’ll need the coordinates for the left, upper, right, and lower boundaries. For example:
- Crop the Image: Use the
crop()
method to crop the image based on the area defined. - View the Cropped Image: You can display the cropped image using
show()
. - Repeat as Needed: You can adjust the coordinates and crop different areas of the same image if needed.
crop_area = (left, upper, right, lower)
cropped_image = image.crop(crop_area)
cropped_image.show()
This process is not only effective but also efficient, allowing you to crop images quickly with minimal code. Next, let’s see how to save and export your cropped images.
Saving and Exporting Cropped Images
Once you have successfully cropped your image, the next step is to save it. Pillow makes this easy with its save()
function. Here’s how to do it:
- Choose a File Name: Decide on a name for your cropped image and the format you want to save it in. For example, you might want to save it as a JPEG or PNG.
- Use the Save Function: Call the
save()
method on your cropped image object. Here’s an example: - Check the Saved Image: Navigate to the directory where you saved the image to ensure it’s there. You can also open it to verify that the cropping was successful.
cropped_image.save("cropped_image.jpg")
File Formats: It’s essential to choose the right file format for your needs:
Format | Use Case |
---|---|
JPEG | Great for photographs with rich colors |
PNG | Best for images needing transparency |
BMP | Good for simple images with less compression |
By following these steps, you can efficiently save and export your cropped images, ensuring they are ready for use in your projects. Happy cropping!
Common Issues and Troubleshooting
While cropping images in Python is generally straightforward, you might encounter a few common issues. Here’s a rundown of these problems and how to solve them:
- File Not Found Error: This often happens when the path to your image is incorrect. Double-check the file name and its location. Make sure you include the right extension.
- Image Display Issues: If the cropped image doesn’t display properly, ensure that you’re using the correct coordinates. It’s easy to mix up the left, upper, right, and lower boundaries.
- Unsupported File Format: If you try to open an image format not supported by Pillow, you’ll get an error. Stick to common formats like JPEG, PNG, or BMP.
- Memory Errors: Large images can consume a lot of memory. If you face memory errors, consider resizing the image before cropping or working with lower-resolution versions.
Troubleshooting Tips:
- Read error messages carefully—they often tell you what went wrong.
- Print the coordinates you’re using for cropping to ensure they make sense.
- Search online forums or documentation for solutions to specific issues you encounter.
By keeping these troubleshooting tips in mind, you’ll be able to tackle most common issues with ease and continue your image cropping adventures!
FAQ
Here are some frequently asked questions about cropping images with Python:
- What is the best format to save cropped images? It depends on your needs. JPEG is good for photographs, while PNG is great for images that need transparency.
- Can I crop multiple images at once? Yes, you can use a loop in Python to iterate through multiple images and apply the same cropping logic.
- Is it possible to undo cropping? Once you save a cropped image, you cannot undo it. Always keep a backup of the original image before cropping.
- Do I need programming experience to crop images with Python? Basic knowledge of Python is helpful, but you can learn as you go with this guide.
- Can I crop images using other libraries? Yes, there are other libraries like OpenCV and scikit-image that can also crop images, but Pillow is user-friendly for beginners.
Conclusion
Cropping images with Python is a valuable skill that opens the door to various image processing tasks. By using the Pillow library, you can easily crop images to focus on what matters most. This guide covered everything from setting up your environment to troubleshooting common issues. Remember, practice makes perfect. The more you experiment with cropping and manipulating images, the more comfortable you will become with Python and its capabilities.
So, grab your favorite images and start cropping away! Whether you’re preparing images for a project, social media, or just for fun, you now have the tools to make your images stand out. Happy coding!