Have you ever wanted a virtual assistant that could have a conversation with you just like a person? Well the ChatGPT API is here to make that happen. Created by OpenAI this tool lets developers incorporate the capabilities of GPT (Generative Pretrained Transformer) into their apps. Picture a chatbot that can grasp context offer helpful replies and even engage in a meaningful dialogue.When I initially delved into the ChatGPT API I was astonished by its ability to interact seamlessly and adapt to different situations. Its akin to chatting with a well informed buddy who’s always on hand.
Getting Started with Python for ChatGPT API
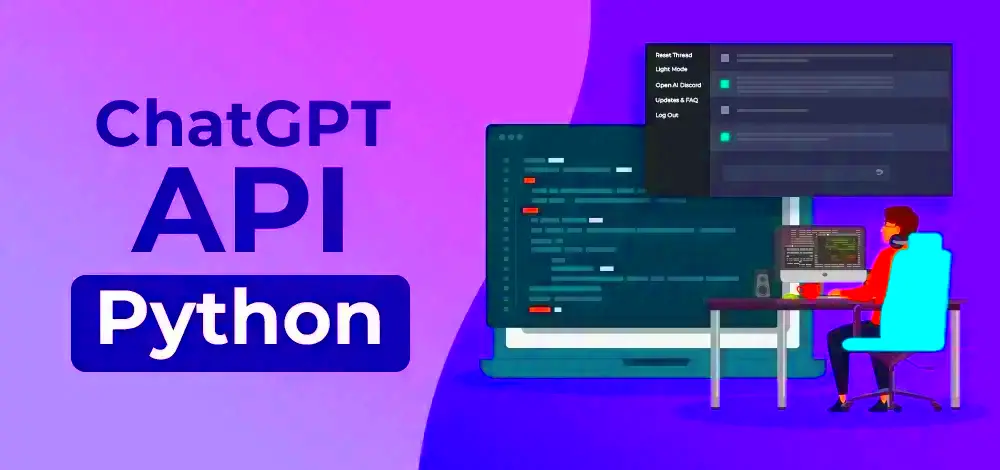
Python is an excellent option when it comes to utilizing the ChatGPT API. Its ease of use and clarity make it suitable for newcomers while still being robust for more experienced users. To kick things off you’ll require some essential tools and libraries. Begin by ensuring that Python is set up on your device. If you haven't done so already you can easily download and install it from the website.Next, you’ll need the
requests library, which is essential for making HTTP requests. You can install it using pip:
pip install requests
After getting Python up and running and installing the required libraries you can jump straight into coding. In the upcoming sections well explore how to establish a connection with the ChatGPT API manage its responses and implement real world scenarios. Its going to be a journey packed with discovery and trial and error similar to my own adventure when I first incorporated this API into a project. The outcomes were remarkable and I cant wait for you to see how it can elevate your applications.
Setting Up Your Environment
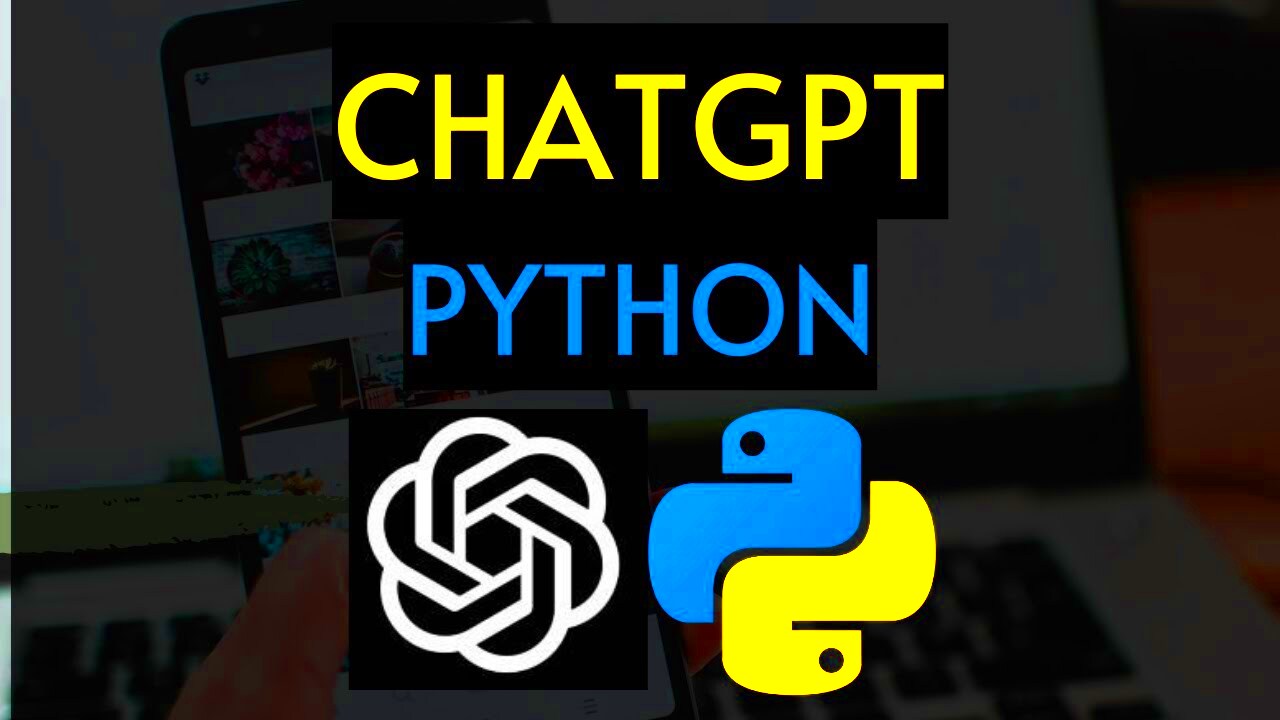
Before jumping into coding its essential to get your environment set up correctly. This step makes sure you have all the necessary tools and settings in place for a seamless development process.Here’s a brief overview to help you set things up smoothly.
- Install Python: Ensure you have the latest version of Python. You can download it from the official Python website.
- Create a Virtual Environment: It’s a good practice to use a virtual environment to manage your project dependencies. You can create one using the following commands:
python -m venv myenv
source myenv/bin/activate # On Windows use: myenv\Scripts\activate
- Install Required Libraries: Besides requests, you might need additional libraries depending on your project. For example:
pip install openai
- Obtain API Key: Sign up on the OpenAI platform and generate your API key. This key will be used to authenticate your requests to the ChatGPT API.
- Configure API Key: For security, store your API key in an environment variable or a configuration file rather than hardcoding it into your scripts.
Getting everything ready might feel like a task but believe me, it pays off. I recall my first time arranging my workspace; it was like getting the stage ready for a spectacular show. Once everything was set up, the coding part felt like the exciting highlight of the performance. So take your time with this setup and you'll be grateful to yourself, for it later on.
Connecting to the ChatGPT API
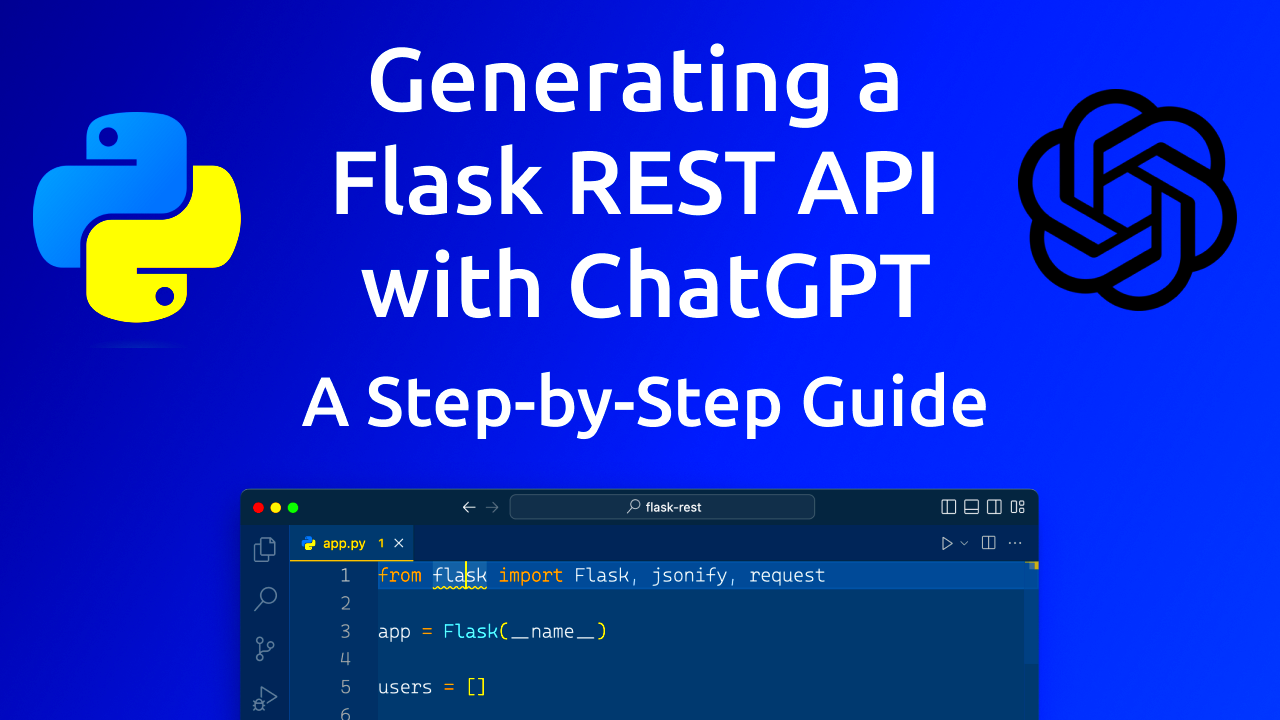
Integrating with the ChatGPT API is akin to unlocking a realm of engaging conversations. My initial connection to the API was akin to stumbling upon an innovative means of engaging with tech. While the process is simple grasping the essentials can prevent any bumps in the road.To connect, follow these steps:
- Prepare Your API Key: You’ll need the API key you obtained from OpenAI. This key is your ticket to access the API.
- Set Up Authentication: Use this key to authenticate your requests. It’s usually added to the header of your HTTP requests. Here’s a basic example:
import requestsheaders = {
'Authorization': 'Bearer YOUR_API_KEY'
}response = requests.get('https://api.openai.com/v1/engines/davinci-codex/completions', headers=headers)
- Make Your First Request: With the authentication in place, you can now make requests to the API. The endpoint you use will depend on the specific functionality you want to access. For a simple text completion, it might look like this:
data = {
'prompt': 'Once upon a time...',
'max_tokens': 50
}response = requests.post('https://api.openai.com/v1/engines/davinci-codex/completions', headers=headers, json=data)
print(response.json())
Establishing a connection with the API was a thrilling milestone in my journey. It felt akin to setting the groundwork for an upcoming venture that would soon materialize. After making that initial connection there’s a satisfaction and eagerness to delve deeper into what’s possible.
Basic Operations with the API
After establishing a connection with the ChatGPT API you can begin utilizing it efficiently. The API provides a range of functions that enable you to create text, finish prompts and engage with your application in versatile manners.Here are some fundamental operations:
- Generate Text: This is the core function. You send a prompt, and the API returns a continuation. For example:
data = {
'prompt': 'The future of technology is...',
'max_tokens': 100
}response = requests.post('https://api.openai.com/v1/engines/davinci-codex/completions', headers=headers, json=data)
print(response.json()['choices'][0]['text'])
- Adjust Parameters: You can customize responses by adjusting parameters like max_tokens (to limit response length), temperature (for randomness), and top_p (for response diversity).
- Handle Context: To create more coherent conversations, you can manage context by maintaining a history of prompts and responses.
Adjusting these operations is like getting to know someone new. Each tweak in the parameters adds a unique touch to the conversation. Take some time to experiment with the settings and discover what suits your preferences.
Handling Responses and Errors
When using the ChatGPT API dealing with responses and errors is essential. Just like in any conversation not everything goes perfectly and being able to navigate these challenges will enhance your overall experience and effectiveness.Here are some tips for managing replies and mistakes in an efficient manner.
- Parsing Responses: The API’s response is typically in JSON format. Make sure to parse this properly to extract useful information:
response_data = response.json()
print(response_data['choices'][0]['text'])
- Managing Errors: Errors can occur for various reasons, from network issues to invalid API keys. Check the HTTP status codes and error messages to diagnose and fix issues:
Status Code | Meaning |
---|
400 | Bad Request - Often due to incorrect parameters |
401 | Unauthorized - Check your API key |
500 | Internal Server Error - Try again later |
- Implementing Error Handling: Use try-except blocks to catch exceptions and handle them gracefully. For example:
try:
response = requests.post('https://api.openai.com/v1/engines/davinci-codex/completions', headers=headers, json=data)
response.raise_for_status() # Raise an error for bad responses
print(response.json())
except requests.exceptions.RequestException as e:
print(f'Error: {e}')
Dealing with these aspects was reminiscent of fixing tech problems during my college days—every challenge brought a lesson. With time you’ll improve in navigating these obstacles and ensuring your interactions with the API are seamless.
Practical Examples and Use Cases
Delving into real world applications and scenarios for the ChatGPT API is akin to discovering an array of seasonings to elevate a meal. Each application highlights a distinct aspect of what this robust tool is capable of. Allow me to present some situations, where the API truly excels.One use case is in
customer support. Imagine integrating ChatGPT into your support system to handle common queries. It can provide quick, consistent responses to frequently asked questions, freeing up human agents for more complex issues. When I first set this up, it was like having an extra pair of hands in the office—handling routine tasks while human staff could focus on more nuanced conversations.Another interesting application is in
content generation. Whether you’re crafting blog posts, generating creative writing, or brainstorming ideas, ChatGPT can be a great co-author. For instance, I once used it to brainstorm topics for a series of blog posts. It provided diverse ideas that I might not have considered, enriching my content strategy.Finally, consider using the API for
personal assistants. Imagine a virtual assistant that can help you schedule meetings, send reminders, or even draft emails. Setting up such a system was a bit like building a personal assistant that knows your preferences and needs. It added a layer of convenience to my daily tasks that I didn’t know I needed until I experienced it.These instances highlight the flexibility of the ChatGPT API. It’s a resource that adjusts to diverse requirements proving to be an asset in a range of situations.
Best Practices for Using the API
To use the ChatGPT API effectively you need to do more than just send requests and get replies. Its about maximizing the API's potential while keeping your application dependable and user friendly. Here are a few tips I've gathered during my journey.
- Optimize Your Prompts: Craft clear and specific prompts to get the best results. The more precise your prompt, the more relevant the response. For instance, instead of asking, “Tell me about technology,” try “Explain how artificial intelligence is transforming healthcare.”
- Manage Token Usage: The API has token limits for requests. To avoid hitting these limits, be mindful of the length of your prompts and responses. Break down large tasks into smaller requests if needed.
- Implement Rate Limiting: To prevent your application from being overwhelmed, implement rate limiting. This will help manage the number of requests sent to the API and avoid potential throttling.
- Handle Errors Gracefully: As discussed earlier, proper error handling is crucial. Make sure your application can handle API errors without crashing and provides meaningful feedback to users.
- Secure Your API Key: Treat your API key like a password. Avoid hardcoding it into your application and use environment variables or secure storage solutions instead.
- Monitor Usage: Keep an eye on your API usage to ensure you stay within your quota. Regular monitoring helps you understand how your application interacts with the API and can highlight areas for improvement.
By integrating these strategies you can improve the functionality of your app and ensure a more seamless user experience. Think of it as tuning an instrument to achieve optimal performance.
FAQ
1. How do I get an API key for ChatGPT?
To get an API key you need to sign up on the OpenAI website. After you register you can create an API key through your account dashboard. This key is essential for verifying your requests to the ChatGPT API.
2. What are the usage limits for the ChatGPT API?
The limits on usage are determined by the subscription plan you have with OpenAI. There are both free and paid options available, each with its own set of quotas and rate limits. For specific details regarding the limitations of your plan you can refer to the OpenAI documentation or check your account information.
3. Can I use the ChatGPT API for commercial purposes?
Absolutely. You’re free to utilize the API for business purposes just be sure to go through OpenAI’s terms of service and acceptable use policies to stay in line with their rules. Staying informed about the guidelines is a wise move to prevent any potential problems later on.
4. What programming languages can I use with the ChatGPT API?
Although this guide primarily emphasizes Python you can utilize the ChatGPT API with any programming language that supports HTTP requests. This encompasses languages such as JavaScript, Ruby, Java and others. The crucial aspect lies in appropriately managing HTTP requests and responses within your selected language.
5. How can I improve the accuracy of responses from the ChatGPT API?
To enhance precision it is important to create prompts that are both and specific. By offering context in your prompts you can assist the API in producing more pertinent and precise replies. Try out various prompt formats to discover what delivers optimal outcomes, for your particular requirements.These frequently asked questions address issues and aim to assist you in using the ChatGPT API more confidently. For inquiries you may also find valuable information by checking OpenAI's documentation or contacting their support team for further assistance.
Conclusion
Diving into the ChatGPT API has been quite an adventure filled with chances to improve your apps and enhance user interactions. From getting your setup ready to getting the hang of API calls every step presents an opportunity to incorporate a tool into your projects. Looking back on my own journey I recall the thrill of making that initial successful request and witnessing the possibilities unfold.Whether you plan to use the API for support, content creation or personal assistance the key lies in grasping its capabilities and following practices. As you embark on your projects remember to keep experimenting and fine tuning your methods. The ChatGPT API is a valuable partner and with careful integration it can significantly enhance your applications conversational features. Cheers to your success in leveraging this remarkable technology!