When building a web application with React, handling images correctly is crucial for both performance and visual appeal. Images, whether stored locally or externally, play an essential role in modern web design. But how do you effectively manage image URLs in React? In this guide, we’ll walk through how to use image URLs in your React components, ensuring they load efficiently and are easily integrated into your app.
Using image URLs in React is quite straightforward, but it’s important to understand the different ways images can be handled. Whether you’re embedding a local image or referencing an external URL, knowing when and how to use
Understanding the Importance of Image URLs in React
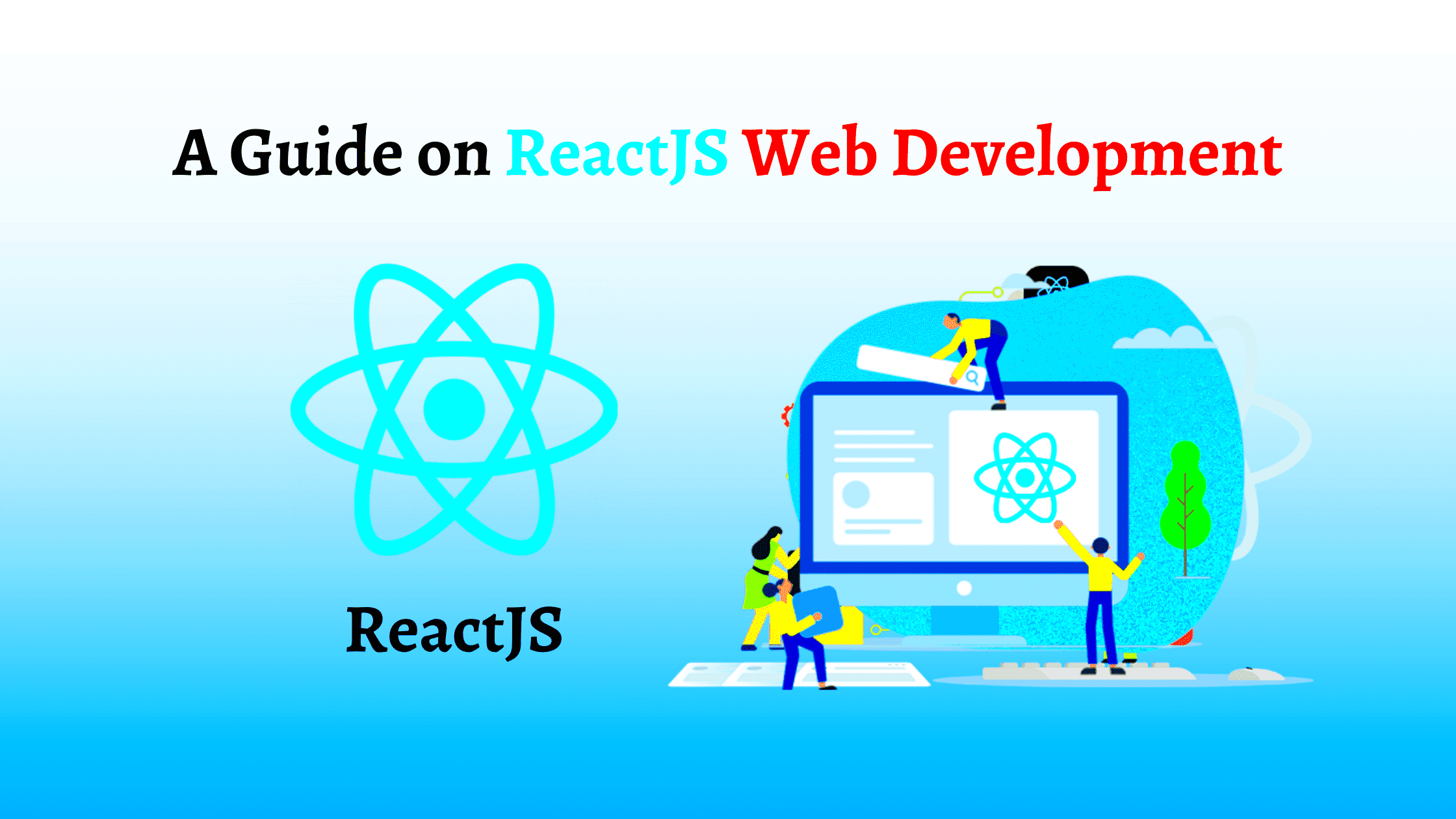
Images are an essential part of web design. They not only enhance the visual appeal of your application but also communicate information and create an engaging user experience. In React, handling image URLs correctly can significantly impact performance, loading times, and SEO.
Here’s why image URLs matter in React:
- Optimized Performance: By using the right image URLs, you can optimize loading times, especially for images hosted externally. React allows for lazy loading, so only images that are currently in view are loaded, improving the overall app speed.
- SEO Benefits: Properly referenced images contribute to better SEO practices. For example, using
alt
text helps search engines understand the content of the images. - Flexible Image Management: React allows you to dynamically manage images using state and props, making it easier to work with images in an interactive app.
- Image Consistency: Storing images in a centralized location and referring to them using URLs ensures that they are consistent across your application.
Also Read This: How to Trace an Image on a Canvas for Art or Design Projects
Steps to Use Image URL in React
Now that you understand the importance of image URLs in React, let’s dive into how to use them in your app. There are several ways to manage and display images using URLs, whether they are stored locally or externally. Below are the steps to follow:
1. Adding Local Images
If you want to use an image that is stored locally in your project, you can import it and then use it in your JSX code. Here’s how:
- Place your image file (e.g.,
my-image.jpg
) in thesrc
folder or an assets directory. - Import the image into your component file:
import MyImage from './path/to/my-image.jpg';
Now, you can reference the image in your JSX code like this:
<img src={MyImage} alt="Description of Image" />
2. Using External Image URLs
For external images, you can simply use the direct URL of the image. You can add this URL directly in the src
attribute of your img
tag. For example:
<img src="https://example.com/path/to/image.jpg" alt="External Image" />
3. Handling Dynamic Image URLs
If the image URL is dynamic (for example, fetched from an API or determined based on user input), you can store the URL in your component’s state or props. This allows you to change the image based on the app’s state or user actions:
const [imageUrl, setImageUrl] = useState('https://example.com/image1.jpg');
Then, use the state variable in your img
tag:
<img src={imageUrl} alt="Dynamic Image" />
These are the basic steps to use image URLs in React. By understanding these methods, you’ll be able to efficiently manage and display images in your web applications.
Also Read This: Shopify Secrets Revealed: Integrating Alibaba into Your Store for Success
Ways to Add Image URLs in React Components
When you’re working with React, there are several ways to add image URLs to your components. Depending on the location of the image (local or external) and how dynamic the image needs to be, you can choose the best method for your needs. Let’s explore the most common ways to handle image URLs in React.
1. Using Local Images with Imports
For images stored in your project, the most straightforward method is to import them. This approach works well when the image is part of your project’s files, and you want to bundle them with your app.
- Place the image in the
src
folder or another folder within your project. - Import the image into the component file like this:
import Logo from './assets/logo.png';
Once the image is imported, you can use it directly in your JSX like this:
<img src={Logo} alt="Website Logo" />
2. Directly Using Image URLs
If the image is hosted online or on an external server, you can simply use the URL in the src
attribute:
<img src="https://example.com/image.jpg" alt="Image from URL" />
3. Using Dynamic Image URLs
If you need to display different images based on user actions or other dynamic data, you can store the image URL in state or props:
const [imageUrl, setImageUrl] = useState('https://example.com/default-image.jpg');
Then, use the variable as the src
value:
<img src={imageUrl} alt="Dynamic Image" />
These are the main ways to add image URLs in React. The method you choose will depend on whether the image is local or external, and whether it needs to be dynamic.
Also Read This: Alibaba to Shopify: Your Ultimate Guide to Selling Success
Handling External Image URLs in React
In many cases, your images may not be stored locally within your React project but are instead hosted externally. This could be on a cloud storage service, your own server, or any other platform that serves images. Handling external image URLs in React is simple, but there are a few best practices to keep in mind to ensure good performance and reliability.
1. Direct URL in img
Tag
If the image is hosted externally, you can reference it directly in the src
attribute of the img
tag. Here’s an example:
<img src="https://example.com/external-image.jpg" alt="External Image" />
This is the simplest way to use external images, as long as the URL is valid and the server hosting the image is reliable.
2. Using External Image URLs Dynamically
Just like with local images, you can also use external URLs dynamically. For example, if the image URL is fetched from an API or determined by user input, you can store it in your React component’s state:
const [imageUrl, setImageUrl] = useState('https://example.com/default-image.jpg');
Then use the imageUrl
in your img
tag:
<img src={imageUrl} alt="Dynamic External Image" />
3. Using Image Caching
One of the challenges with external images is ensuring that they load quickly. You can implement caching strategies to avoid repeatedly fetching the same images. For example, using a CDN (Content Delivery Network) can speed up image loading times by serving the image from a server that’s geographically closer to the user.
Handling external images in React is straightforward, but it's important to consider performance and reliability. Use dynamic URLs and caching to optimize the user experience.
Also Read This: how to install iso image
Best Practices for Using Image URLs in React
When working with images in React, following best practices ensures your app remains fast, efficient, and easy to maintain. Below are some key practices to consider when using image URLs in your React components:
1. Optimize Image Size
Large image files can slow down your app, so always optimize images before uploading them. Tools like ImageOptim and TinyPNG can help reduce file sizes without compromising quality.
2. Use Lazy Loading
Lazy loading only loads images when they are visible on the screen. This can significantly improve the initial loading time of your app, especially if you have many images. To implement lazy loading in React, you can use the loading="lazy"
attribute in your img
tags:
<img src="image.jpg" alt="Lazy Loaded Image" loading="lazy" />
3. Add Alt Text for Accessibility
Adding descriptive alt
text to images not only improves SEO but also makes your app more accessible to users with visual impairments. Always include alt text in your img
tags:
<img src="image.jpg" alt="A description of the image" />
4. Manage External Images Efficiently
If you’re using external images, make sure they are hosted on reliable servers and consider using a CDN for faster delivery. Also, make sure the image URLs are correct to avoid broken image links.
5. Use Image Placeholders
Before an image loads, it’s a good practice to show a placeholder image or a loading spinner. This provides a better user experience, especially for slower internet connections.
By following these best practices, you’ll ensure that your React app handles images efficiently, which leads to a better user experience and improved performance.
Also Read This: How to Rotate Images in OneNote for Better Layouts
Common Issues with Image URLs in React
While working with image URLs in React, developers often run into a few common issues that can affect the performance or display of images. Understanding these challenges can help you troubleshoot and resolve problems quickly, ensuring that your application functions as expected. Let’s explore some of the most frequent issues you may face when working with image URLs in React.
1. Broken Image Links
One of the most common issues is broken image links, which occur when the image URL is incorrect or the image is missing from the specified location. This can happen with both local and external images. To prevent broken images:
- Ensure the file path is correct when referencing local images.
- Check if the external URL is valid and points to an existing image.
- Use the browser’s developer tools to inspect broken links and resolve the issue.
2. Incorrect Image Format or Type
Another issue is using the wrong image format. React can display various image types, such as JPG, PNG, and GIF, but if you try to load an unsupported format, the image might not display properly. Always check the format and ensure the image type matches your intended use case.
3. Slow Image Load Times
When working with external image URLs, slow loading times can become a problem, especially if the image is hosted on a server far from your users. To improve image load times:
- Use a Content Delivery Network (CDN) for faster delivery.
- Implement lazy loading to only load images when they’re needed.
4. Caching Issues
Sometimes, images may not update correctly due to caching. If you update an image but the old version is still being displayed, try clearing your cache or force-refreshing the page to see the latest image.
By understanding these common issues and their solutions, you can more easily troubleshoot problems with image URLs in React and ensure smooth image display throughout your application.
Also Read This: Cart Command: Adding Items to Your Cart on AliExpress
FAQ
In this section, we’ll answer some frequently asked questions about using image URLs in React. Whether you’re new to React or have been using it for a while, these answers will help clarify any doubts you may have.
1. Can I use a relative image URL in React?
Yes, you can use relative image URLs in React, especially for images stored locally in your project. Simply import the image and use it in your component, as shown earlier in the guide.
2. How do I add dynamic images in React?
To add dynamic images, you can store the image URL in your component's state and update it based on user actions or other dynamic data. For example:
const [imageUrl, setImageUrl] = useState('https://example.com/image1.jpg');
3. How can I improve the performance of images in React?
To improve the performance of images in React, consider optimizing the image sizes before uploading them, using lazy loading, and employing a CDN for external images. Also, make sure to use the correct format for each image type.
4. What is lazy loading and how do I use it in React?
Lazy loading is a technique that only loads images when they are visible on the screen. In React, you can implement lazy loading by adding the loading="lazy"
attribute to your img
tags:
<img src="image.jpg" alt="Lazy Loaded Image" loading="lazy" />
Conclusion
In conclusion, using image URLs in React is a powerful way to manage and display images in your web applications. Whether you’re working with local images or fetching external ones, understanding the different methods and best practices will help you optimize the user experience and improve app performance. Don’t forget to consider issues like broken links, slow loading times, and caching, and address them with simple solutions like lazy loading and using a CDN.
By following the steps and guidelines outlined in this guide, you’ll be well on your way to mastering image URLs in React. With careful management and optimization, you can ensure that your images are displayed quickly and correctly, providing a smooth experience for your users.