Displaying images in Python is a common task for anyone working with visual data, machine learning models, or graphical applications. Whether you're visualizing data, building a computer vision model, or creating a graphical user interface, showing images can be essential to your project. Python has a number of libraries that make it easy to display images with just a few lines of code.
In this blog post, we will walk through different methods for displaying images in Python using popular libraries. We'll look at how to work with these libraries, the advantages of each, and how to handle different image formats. Whether you're a beginner or experienced with Python, you'll find useful tips and tricks to make displaying images easier.
Choosing the Right Library for Image Display
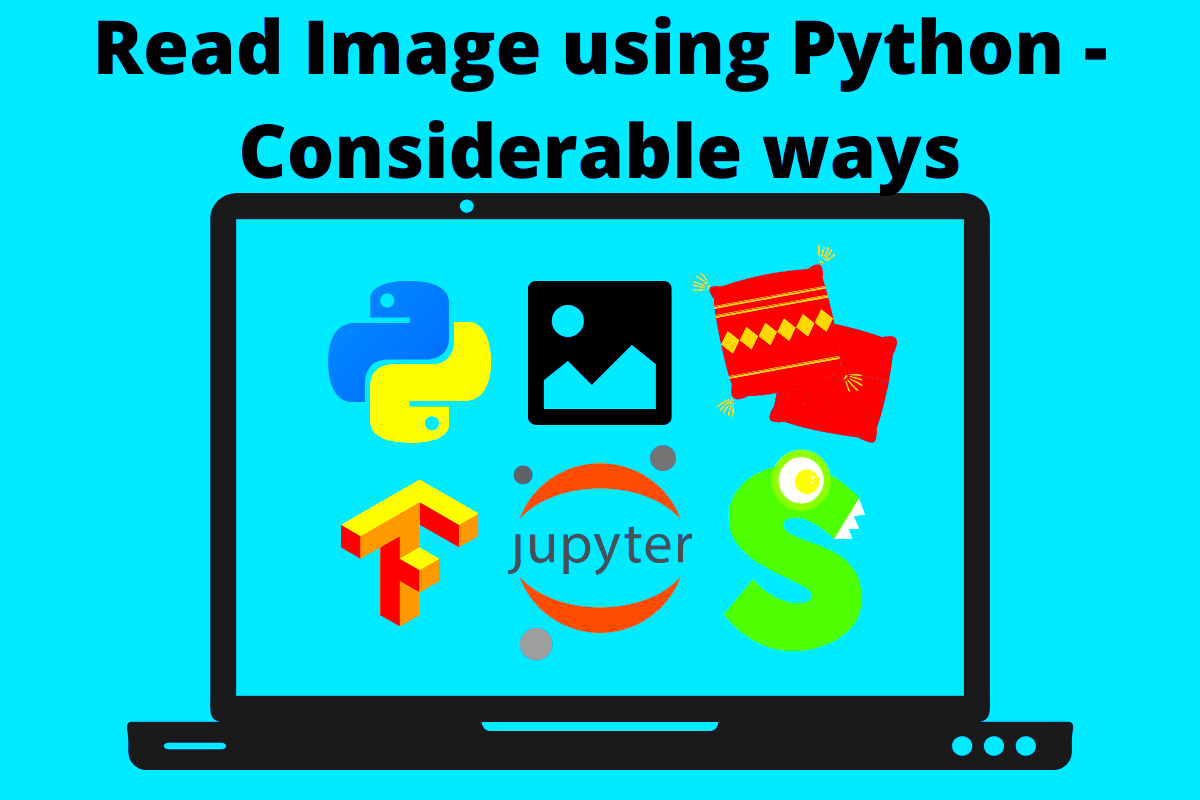
When it comes to displaying images in Python, choosing the right library depends on your project requirements. Some libraries are simple and focused on just displaying images, while others offer additional features like image manipulation or complex GUI integration. Here's a comparison of some popular libraries:
- Matplotlib: Primarily used for data visualization, it’s a great choice for quickly displaying images as part of data analysis tasks.
- Pillow (PIL): A powerful library for image manipulation, ideal for more control over image processing and display.
- OpenCV: A comprehensive library for computer vision, perfect for applications that require advanced image processing and manipulation.
- Tkinter: If you’re working on a GUI application, Tkinter integrates well for displaying images alongside other UI components.
Consider the complexity of your project. If you need simple image display, Matplotlib or Pillow is enough. However, for more complex tasks, OpenCV or Tkinter might be better suited.
Using Matplotlib for Displaying Images
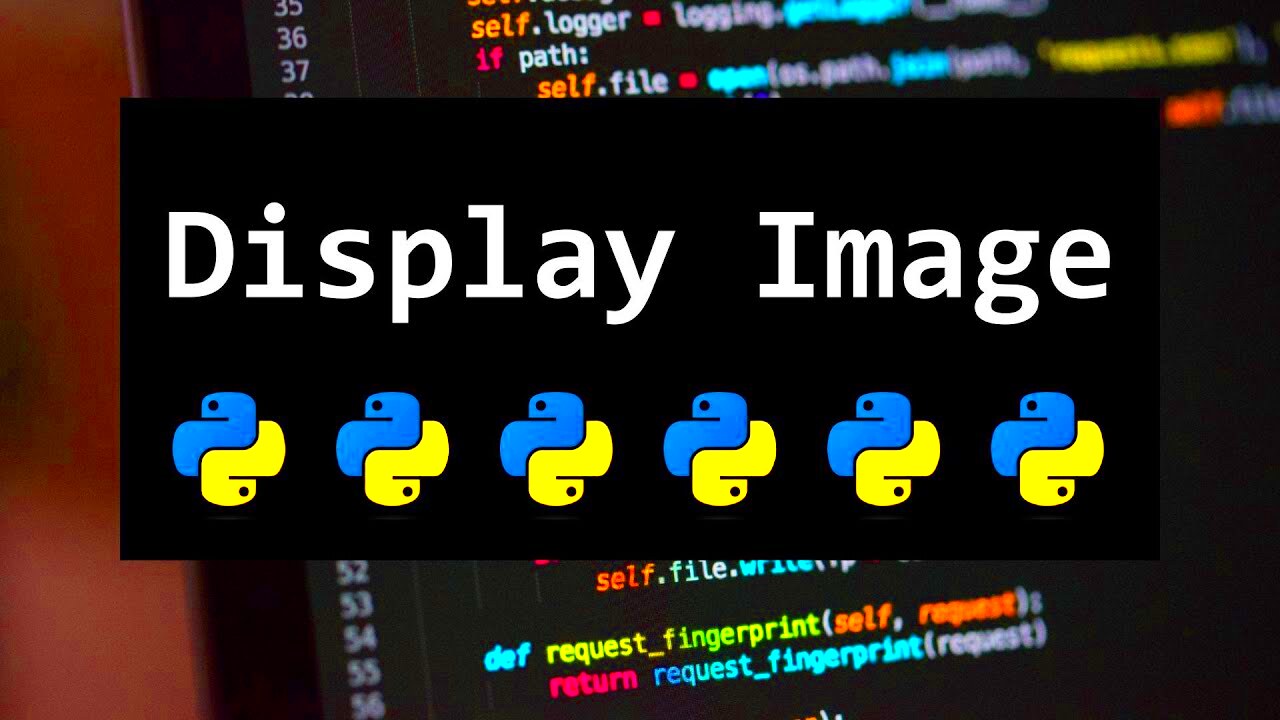
Matplotlib is a widely used library for data visualization, but it also provides a simple way to display images. It’s great for tasks like showing images within the context of data analysis or creating visualizations in scientific computing. Here's how to use it for image display:
To get started with Matplotlib, you’ll need to install it first if you don’t have it already. You can do this using the command:
pip install matplotlib
Once installed, displaying an image is simple. Here's a basic example:
import matplotlib.pyplot as plt
import matplotlib.image as mpimg
# Load an image
img = mpimg.imread('path_to_image.jpg')
# Display the image
plt.imshow(img)
plt.show()
In this example, imread()
reads the image, and imshow()
displays it. The show()
function ensures that the image is rendered to the screen.
One of the advantages of using Matplotlib is that it allows for easy customization. You can adjust the image size, add titles, labels, and even overlay plots on the image, making it highly flexible for data analysis tasks.
Pros of Using Matplotlib | Cons of Using Matplotlib |
---|---|
Simple to use for basic image display | Not ideal for image manipulation |
Great for integrating images into data visualizations | Limited in terms of advanced image processing |
Matplotlib is excellent for displaying static images in data-driven projects. However, if you need more control over the image or need to manipulate it, you might consider using other libraries like Pillow or OpenCV.
Using PIL or Pillow for Image Display
Pillow, which is the modern version of the Python Imaging Library (PIL), is an excellent library for image manipulation and display. It's very popular among Python developers because of its simplicity and versatility. While Matplotlib is great for visualizing data, Pillow shines when you need more control over how images are processed and displayed.
To get started with Pillow, you first need to install the library:
pip install pillow
Once installed, displaying an image is simple. Here’s an example of how to load and display an image using Pillow:
from PIL import Image
# Open an image file
img = Image.open('path_to_image.jpg')
# Display the image
img.show()
The open()
method loads the image, and the show()
method opens it in the default image viewer. This is perfect for quick and straightforward image display tasks.
While Pillow is known for its ease of use, it also offers a wide range of features, such as:
- Resizing and cropping images
- Rotating and flipping images
- Applying filters and effects
- Saving images in various formats like PNG, JPEG, and BMP
Overall, Pillow is a great choice if you need to display and manipulate images quickly. It’s lightweight and highly efficient, making it suitable for both simple tasks and more advanced image processing.
Pros of Using Pillow | Cons of Using Pillow |
---|---|
Easy to use for basic image tasks | Limited for complex image processing compared to OpenCV |
Supports a wide range of formats | Less suitable for interactive image display |
Using OpenCV for Advanced Image Display
OpenCV (Open Source Computer Vision Library) is one of the most powerful libraries available for real-time computer vision tasks. It’s widely used in fields like robotics, machine learning, and image processing. OpenCV excels in handling large-scale image data, making it the go-to choice for more complex image display requirements, especially when you need to work with video feeds or real-time image processing.
To use OpenCV for displaying images, you first need to install the library:
pip install opencv-python
After installation, here’s a basic example of how to read and display an image:
import cv2
# Read the image
img = cv2.imread('path_to_image.jpg')
# Display the image
cv2.imshow('Image', img)
# Wait for a key press and close the window
cv2.waitKey(0)
cv2.destroyAllWindows()
In this example, imread()
loads the image, and imshow()
displays it in a window. The waitKey()
function waits for a key press to close the window, and destroyAllWindows()
closes the window after the key press.
OpenCV’s main strengths include:
- Real-time image and video processing
- Advanced features like object detection, face recognition, and more
- Support for various image formats and video streams
While OpenCV offers powerful tools for advanced image processing, its complexity makes it less suitable for basic tasks like simple image display. If you're working on a basic project, a simpler library like Pillow might be a better fit.
Pros of Using OpenCV | Cons of Using OpenCV |
---|---|
Powerful for advanced computer vision tasks | More complex to use for simple image display |
Real-time image and video processing support | Requires more resources for large-scale processing |
Displaying Images with Tkinter for GUI Applications
Tkinter is Python’s standard library for creating graphical user interfaces (GUIs). While Tkinter is not specifically designed for image display, it integrates well with libraries like Pillow to display images in your applications. If you're building a desktop application and need to show images as part of the interface, Tkinter is a great choice.
To get started with displaying images in Tkinter, you’ll need both Tkinter and Pillow. Here’s a simple example:
from tkinter import *
from PIL import Image, ImageTk
# Create a Tkinter window
root = Tk()
# Open an image file
img = Image.open('path_to_image.jpg')
# Convert the image for Tkinter compatibility
img_tk = ImageTk.PhotoImage(img)
# Create a label widget with the image
label = Label(root, image=img_tk)
label.pack()
# Run the Tkinter event loop
root.mainloop()
This example uses Tkinter to create a window and a label widget to display the image. The ImageTk.PhotoImage()
method is used to convert the image into a format that Tkinter can understand.
Using Tkinter has the advantage of allowing you to build interactive GUI applications where you can display images alongside other UI components like buttons, sliders, and text fields. It’s a great choice for building desktop applications.
- Easy integration with other GUI components
- Provides an event-driven programming model
- Good for building desktop applications
However, if you’re not building a GUI and just need to display images quickly, Tkinter might be overkill compared to simpler libraries like Matplotlib or Pillow.
Pros of Using Tkinter | Cons of Using Tkinter |
---|---|
Good for building desktop applications | Not the best for simple image display tasks |
Easy to integrate with other UI components | Limited image processing capabilities |
Handling Different Image Formats in Python
When working with images in Python, it's essential to understand how to handle various image formats. Images come in different types, such as PNG, JPEG, GIF, BMP, and TIFF, each with its own characteristics. Whether you're working with web data, creating machine learning datasets, or developing GUI applications, knowing how to load, save, and convert between these formats is key to handling images effectively.
In Python, libraries like Pillow, OpenCV, and Matplotlib can help you work with different image formats. Here's a breakdown of the most common formats:
- PNG (Portable Network Graphics): This format supports lossless compression and transparency, making it ideal for images with clear edges and graphics.
- JPEG (Joint Photographic Experts Group): A popular format for photographs, JPEGs offer lossy compression, which reduces file size but may degrade image quality.
- GIF (Graphics Interchange Format): GIF supports animations and is commonly used for simple graphics with limited color depth.
- BMP (Bitmap): This format is uncompressed and can result in large file sizes. It's not widely used today but can be useful for some legacy applications.
- TIFF (Tagged Image File Format): TIFF is a flexible format, often used in professional photography and scanning due to its support for high-quality images.
With libraries like Pillow, you can easily convert images from one format to another, using the save()
function. Here's an example of saving a PNG image as a JPEG:
from PIL import Image
img = Image.open('image.png')
img.save('image.jpg', 'JPEG')
Understanding the nuances of different image formats will help ensure that your applications handle image data efficiently and with minimal quality loss.
Common Image Formats | Use Cases |
---|---|
PNG | Good for graphics, transparent images, and web use |
JPEG | Ideal for photographs and web images where file size is important |
GIF | Best for simple animations and low-quality images |
BMP | Used for uncompressed images with large file sizes |
TIFF | Used for high-quality images in scanning and professional photography |
Common Issues and How to Fix Them
When displaying or manipulating images in Python, you may encounter several common issues. Fortunately, most of these problems are easy to solve once you understand the underlying causes. Here are some common issues and their solutions:
- Image Not Displaying Properly: This can happen if the image format is unsupported or if the file is corrupted. Always ensure the image is in a compatible format (like PNG or JPEG), and check the file for corruption by opening it in a standard image viewer.
- Incorrect Image Size: If the image doesn't appear at the correct size, it might be due to mismatched aspect ratios or resolution issues. You can resize an image using the
resize()
method in Pillow:
img = img.resize((width, height))
By identifying the root cause of an issue and using the right library or technique, you can quickly resolve most problems when working with images in Python.
Common Issues | Solutions |
---|---|
Image Not Displaying Properly | Check the file format and ensure the image is not corrupted |
Incorrect Image Size | Resize the image using the resize() method in Pillow |
File Not Found | Verify the file path, or use absolute paths |
Image Display Lag | Resize large images or use OpenCV for better performance |
Frequently Asked Questions
Here are some common questions that come up when working with image display and manipulation in Python:
- Q: What is the best library for displaying images in Python?
A: It depends on your needs. If you want simple image display, Matplotlib or Pillow are great options. For advanced image processing, OpenCV is ideal. - Q: Can I display multiple images at once?
A: Yes! You can display multiple images in separate windows using OpenCV'simshow()
function or arrange them side by side using Matplotlib. - Q: How do I handle transparency in images?
A: For images with transparency, use the PNG format. Pillow can help you manipulate and display transparent images by using an alpha channel. - Q: How can I work with animated images (GIFs)?
A: GIFs can be opened and displayed using Pillow, but for more advanced handling of animated images, you might want to use a library like OpenCV. - Q: Can I save an image in a different format?
A: Yes, you can easily convert and save images in a different format using thesave()
method in Pillow, like saving a PNG image as a JPEG.
These answers should help guide you as you work with images in Python, whether you're displaying them for analysis, building an app, or processing large datasets.
Conclusion
In conclusion, displaying and manipulating images in Python is a straightforward task thanks to the powerful libraries available, such as Matplotlib, Pillow, OpenCV, and Tkinter. Each library serves different purposes, and selecting the right one depends on the complexity of your project. For simple image display tasks, libraries like Matplotlib and Pillow are quick and easy to use. If you need more advanced image processing, OpenCV provides a wealth of features for tasks like object detection and real-time video processing. Additionally, Tkinter is ideal for integrating images into graphical user interfaces for desktop applications.
Remember, handling different image formats and solving common display issues requires understanding the image types and the tools at your disposal. With the tips and examples shared in this guide, you should be well-equipped to work with images in Python and handle challenges efficiently. By using the right libraries and methods, you can streamline your image display tasks and focus on more important aspects of your project.