Pulling Docker images is a crucial part of working with Docker. If you want to use a pre-configured environment for your projects, you can easily pull an image from a Docker registry like Docker Hub. These images can contain everything from code and libraries to settings and configurations, making it simpler to set up and run applications in a containerized environment.
Docker images are essentially the blueprint for containers, and pulling them is the first step in getting started with Docker. In this section, we’ll explain the importance of Docker images and how they fit into the broader picture of containerization. Whether you’re a beginner or looking to streamline your workflows, understanding this process is essential.
What is a Docker Image and Why is it Important?
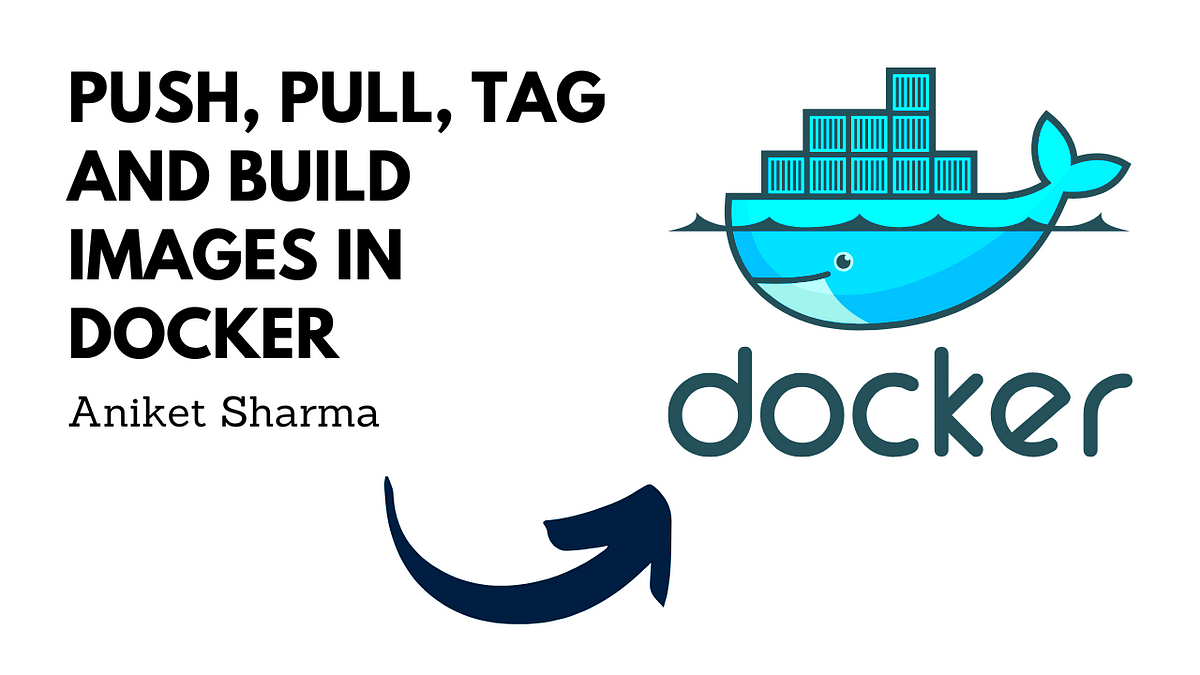
A Docker image is a lightweight, standalone, and executable package that includes everything needed to run a piece of software: the code, runtime, libraries, environment variables, and configurations. Think of it like a snapshot of a specific environment you want to replicate across different systems.
Here’s why Docker images are important:
- Consistency Across Environments: Docker images ensure that your application will run the same way, no matter where you deploy it. Whether it’s on a developer’s local machine, a test server, or a cloud environment, the image contains everything required to ensure consistency.
- Portability: Once you pull a Docker image, you can use it across multiple platforms and architectures without worrying about compatibility issues.
- Efficiency: Docker images are built to be small and shareable, which reduces overhead and makes it easier to distribute software without having to worry about missing dependencies or configurations.
Overall, Docker images simplify the deployment process and ensure that your application works as expected across all environments. They are essential for building reliable, scalable applications with Docker.
Steps to Pull a Docker Image
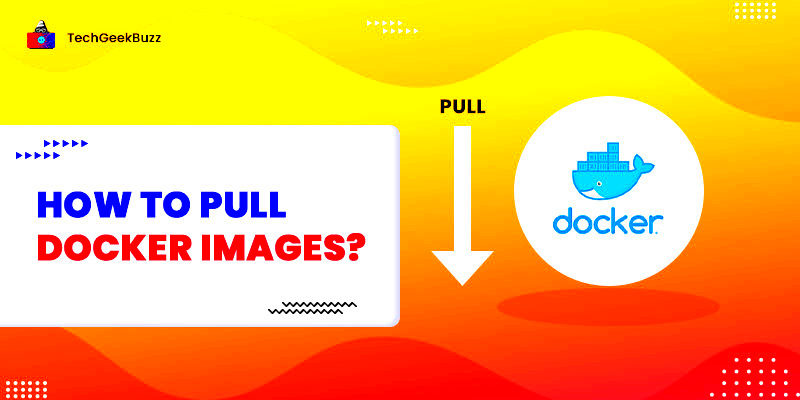
Pulling a Docker image is a straightforward process, but there are a few steps you need to follow to ensure everything works smoothly. Here’s a simple breakdown:
- Step 1: Install Docker – Before you can pull a Docker image, you need to have Docker installed on your system. You can download it from the official Docker website and follow the installation instructions for your operating system.
- Step 2: Log in to Docker Hub (Optional) – If you’re pulling an image from Docker Hub, you can log in to your Docker account using the command
docker login
. This step is optional but recommended if you want to pull private images. - Step 3: Find the Image You Need – Visit the Docker Hub website or search using the
docker search
command to find the image you want. For example, if you need a Python image, search for “python” to find the official Python Docker image. - Step 4: Pull the Image – To pull an image, use the
docker pull
command followed by the image name. For example:docker pull python:3.8
. This will pull the Python 3.8 image from Docker Hub and download it to your local machine. - Step 5: Verify the Image – Once the image has been pulled, you can verify it by listing all the downloaded images with the command
docker images
. You should see the image you just pulled in the list.
And that’s it! Now you’re ready to start using the pulled Docker image for your project. If you run into any issues, make sure to check Docker’s official documentation for more troubleshooting tips.
Understanding Docker Commands for Pulling Images
Docker commands are simple yet powerful tools that allow you to manage and interact with Docker images and containers. When it comes to pulling images, the most common and essential command is docker pull
. This command enables you to download a specific image from a registry like Docker Hub to your local system. Understanding how to use this command and its variations will help streamline your Docker workflows.
Here’s an overview of some key Docker commands related to pulling images:
- docker pull <image-name>: The basic command to pull an image from a Docker registry. For example,
docker pull ubuntu
pulls the latest Ubuntu image. - docker pull <image-name>:<tag>: If you need a specific version of an image, you can specify the tag. For example,
docker pull python:3.8
pulls the Python 3.8 image. - docker search <image-name>: If you don’t know the exact image name, use the search command to look for available images. For example,
docker search node
will list all images related to Node.js. - docker images: After pulling an image, use this command to list all images downloaded to your local machine.
- docker rmi <image-id>: If you want to remove an image, use the
docker rmi
command followed by the image ID.
By mastering these commands, you’ll be able to quickly pull, list, and manage Docker images with ease, setting yourself up for a more efficient development and deployment process.
How to Use Docker Images After Pulling
Once you’ve successfully pulled a Docker image, the next step is to use it in your projects. Docker images are like blueprints that allow you to run applications inside containers. This section will guide you through how to use those images after pulling them.
Here’s how you can get started:
- Running the Image: After pulling an image, the most common action is to run it as a container. To do this, use the
docker run
command. For example,docker run python:3.8
will run the Python 3.8 image in a new container. - Running a Container in Interactive Mode: If you need to interact with the container, you can run it in interactive mode using the
-it
flags. For example:docker run -it ubuntu
will open an interactive terminal session within the Ubuntu container. - Mapping Ports: If your containerized application needs to interact with the outside world (like a web server), you can map container ports to host machine ports. Use the
-p
flag to do this. Example:docker run -p 8080:80 nginx
maps port 80 inside the container to port 8080 on your local machine. - Running Containers in Detached Mode: If you don’t want your terminal to be occupied by the running container, use the
-d
flag. This will run the container in the background. Example:docker run -d nginx
. - Accessing Container Files: You can also run commands inside the container, like accessing the filesystem or installing additional packages. Use
docker exec
to run commands inside an active container. Example:docker exec -it container_id bash
will give you access to the container’s bash shell.
By running and interacting with containers, you can make the most of Docker images and integrate them into your project setup. Containers provide a flexible and efficient way to test, develop, and deploy applications.
Best Practices for Using Docker Images in Your Projects
Docker images are a powerful tool, but to get the most out of them, it’s important to follow some best practices. By adhering to these guidelines, you can ensure that your images are efficient, secure, and easy to manage.
Here are some best practices to keep in mind:
- Use Official Images: Whenever possible, pull official images from Docker Hub or trusted repositories. Official images are maintained by the Docker team or the software’s creators, ensuring they are up-to-date and secure.
- Keep Images Small: Large images take up more disk space and take longer to download. Minimize the size of your Docker images by starting from a minimal base image and only including the dependencies your project needs.
- Tag Images Properly: Always tag your images with meaningful version numbers or descriptive names. This makes it easier to track and manage different versions of the image. For example, use
python:3.8
instead of justpython
to avoid pulling the latest, potentially unstable version. - Regularly Update Images: Docker images can become outdated as new versions of libraries, dependencies, and security patches are released. Regularly update your images to stay secure and take advantage of the latest features.
- Use Docker Compose for Complex Projects: If you’re working with multiple containers, use Docker Compose to define and run multi-container applications. This tool makes it easier to configure and manage dependencies across multiple services in a project.
- Minimize Privileged Containers: Avoid running containers with unnecessary privileges, especially if you’re working with sensitive data. Running containers as non-root users helps improve security and minimize potential vulnerabilities.
- Clean Up Unused Images: Over time, old and unused images can accumulate on your system. Use the
docker image prune
command to remove unused images and free up disk space.
By following these best practices, you’ll be able to optimize your Docker workflow, create more efficient images, and maintain a secure and manageable environment for your projects.
Troubleshooting Common Issues with Docker Images
While working with Docker images, you might run into some common issues. These can range from problems during the image pull process to issues when running containers. The good news is that most problems can be fixed with a bit of troubleshooting. In this section, we'll look at some of the most frequent problems users face and how to resolve them.
Here are a few common issues and solutions:
- Problem 1: Image Pull Fails
One of the most common issues is when thedocker pull
command fails to download an image. This can happen due to network issues, incorrect image names, or private image permissions. To troubleshoot, check your internet connection, ensure the image name is correct, and log in to Docker Hub if the image is private usingdocker login
. - Problem 2: Insufficient Disk Space
Docker images can take up significant space. If you get an error about insufficient disk space, you may need to clear unused images. Usedocker image prune
to remove dangling or unused images. - Problem 3: Container Fails to Start
If your container doesn’t start after pulling an image, it could be due to missing dependencies or improper configurations. Check the logs withdocker logs <container_id>
to identify errors and make necessary adjustments to your Dockerfile or configuration files. - Problem 4: Version Conflicts
Sometimes, version mismatches between images and dependencies can cause issues. To solve this, always specify the correct version tag when pulling an image. For example, usedocker pull node:14
instead of justdocker pull node
. - Problem 5: Permissions Issues
Docker may sometimes face permission issues, especially when accessing files or volumes. Running Docker commands withsudo
may resolve these issues, or you may need to adjust file permissions on your system.
These are just a few examples of the common issues you might encounter with Docker images. Docker provides great logging and error messages to help pinpoint the issue, so don’t hesitate to dive deeper into the logs or consult Docker’s official documentation for further guidance.
Conclusion and Final Thoughts on Docker Images
Docker images are powerful tools that simplify the development, deployment, and scaling of applications. They offer a consistent and portable environment, making it easier to share software across different systems and teams. By pulling Docker images, you can save time by using pre-configured environments instead of setting everything up manually.
To make the most of Docker images, it’s essential to understand how to pull, use, and manage them effectively. By following best practices, such as using official images, keeping images small, and managing versioning, you can build a robust and efficient development workflow. Docker also offers tools like Docker Compose to handle complex setups involving multiple containers, adding another layer of flexibility.
Ultimately, Docker images are a key component of containerization, and mastering them will significantly improve your development and deployment processes. With the right troubleshooting skills and best practices in place, you’ll be able to take full advantage of Docker’s capabilities, making your projects more scalable, reliable, and easier to manage.
FAQ About Pulling and Using Docker Images
Here are some frequently asked questions (FAQs) to help you better understand Docker images and how to pull and use them effectively:
- Q: What is the difference between a Docker image and a Docker container?
A Docker image is a read-only template that defines how a container should be created. A Docker container, on the other hand, is a running instance of an image that includes the application and its dependencies. - Q: Can I pull an image from a private registry?
Yes, you can pull images from a private registry. You’ll need to log in to the registry first using thedocker login
command and provide your credentials to access the image. - Q: How do I remove an image after using it?
To remove an image from your local machine, use thedocker rmi <image-id>
command. This is especially useful when cleaning up unused images to free up disk space. - Q: How can I specify a version when pulling an image?
When pulling an image, you can specify a version by adding a tag. For example,docker pull python:3.8
will pull version 3.8 of the Python image. - Q: What should I do if the image fails to run?
If the image fails to run, check the logs usingdocker logs <container-id>
. This can give you details on what went wrong. Common issues include missing dependencies, permission errors, or incorrect configurations. - Q: How can I see which images are available on my system?
You can use thedocker images
command to list all the images available on your system, including the tags and sizes of each image.
If you have additional questions or need more specific help, Docker’s official documentation is a great resource to explore.