Docker has transformed how we develop and deploy applications. One of its most powerful features is the ability to use Docker images, which are lightweight, portable, and customizable containers that allow developers to package software and its dependencies. Editing Docker images can be a critical step in modifying an application or updating its environment without needing to start from scratch. Whether you're tweaking configurations, installing new software, or fixing bugs, understanding how to edit Docker images can save you time and effort.
Understanding Docker Images and Containers
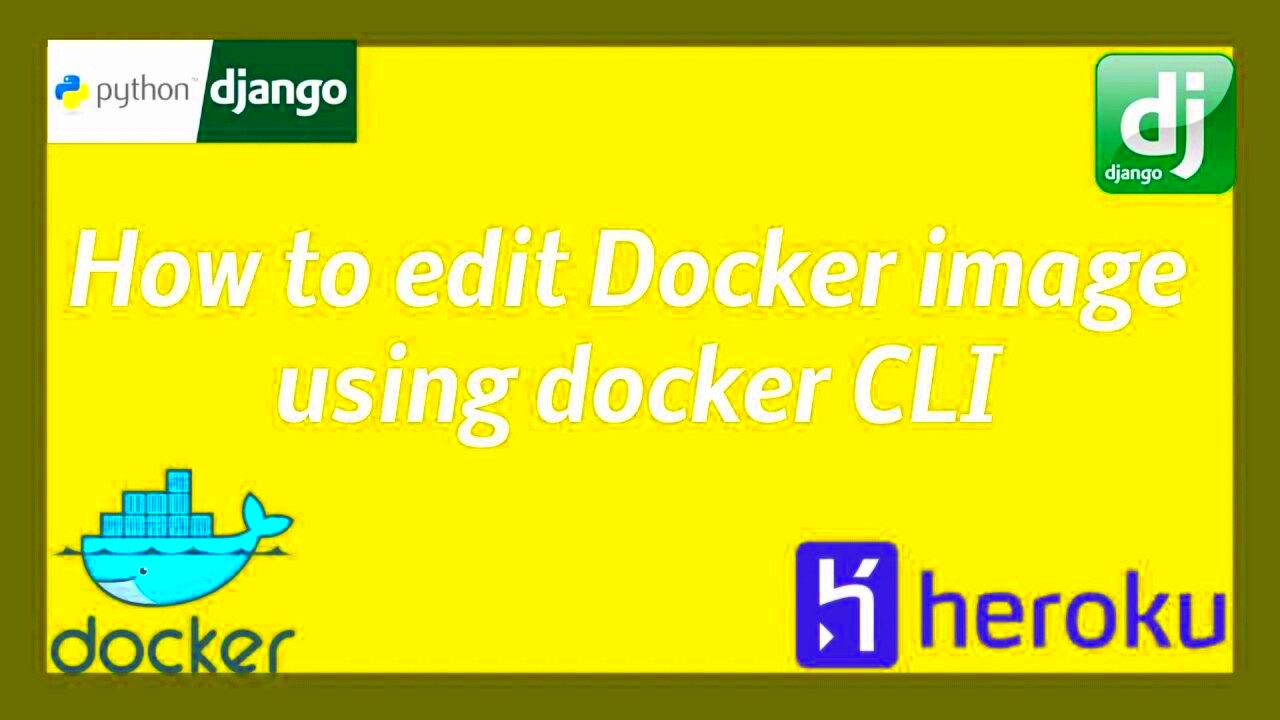
Before diving into editing Docker images, it's important to understand what they are and how they work. Docker images are read-only templates that contain the instructions for creating a container. A container, on the other hand, is a running instance of an image—essentially, it's a virtualized environment that runs your application or service. Let's break it down:
- Docker Images: These are the blueprints that define the environment in which an application will run. They contain everything from the operating system to application libraries and configurations.
- Docker Containers: Containers are created from images and are where the application actually runs. They are isolated from each other and the host system, ensuring that they don't interfere with one another.
In simple terms, Docker images are the templates, and containers are the live, running instances of those templates. When you edit a Docker image, you're essentially altering the template that will be used to generate containers in the future.
Also Read This: How to Train Your Dragon: Iconic Images from the Movie
Why You Might Need to Edit a Docker Image
There are several reasons why you may want to edit a Docker image. Here are some common scenarios:
- Updating the Software: If you need to update an application or library within the image, editing the Docker image allows you to modify the environment without rebuilding everything from scratch.
- Adding New Features: When new features or tools are required in the application, you may need to edit the image to include those changes.
- Optimizing Performance: By editing an image, you can fine-tune configurations or remove unnecessary components to make the image more lightweight and efficient.
- Fixing Bugs: If there are bugs or security vulnerabilities in the current Docker image, editing it allows you to patch those issues directly within the image.
In many cases, editing the Docker image can help speed up development and testing. Instead of manually updating containers each time you need a change, editing the base image ensures that all new containers created from it will automatically reflect the changes you’ve made.
Also Read This: How to Sell Photos on Getty Images
Prerequisites for Editing Docker Images
Before you start editing Docker images, you need to make sure you have the right tools and environment set up. Fortunately, the prerequisites are straightforward and easy to handle, as long as you have a basic understanding of Docker. Here's what you'll need:
- Docker Installed: You need Docker installed on your system. Docker is available for most operating systems, including Linux, Windows, and macOS. If you haven't installed it yet, head over to the official Docker website to download the version for your OS.
- Basic Knowledge of Docker Commands: Understanding Docker commands like
docker pull
,docker run
,docker build
, anddocker commit
is crucial for editing images effectively. If you're new to Docker, it's a good idea to familiarize yourself with these basic commands first. - Access to a Base Image: To edit an image, you'll need to either use an existing image or create one. If you're starting from an existing image, make sure you know which one you're working with. Popular base images include official images like
ubuntu
,node
, andpython
. - Text Editor or IDE: You’ll likely need a text editor (like VS Code or Sublime Text) to modify configuration files or write Dockerfiles for building new images. An integrated development environment (IDE) can also be helpful if you're working on more complex projects.
- Docker Hub Account (Optional): If you plan to share your edited Docker images, having a Docker Hub account allows you to push your images to the cloud. This isn't strictly required for local image editing but is helpful for collaboration and storage.
Once you have these prerequisites in place, you can start editing Docker images with confidence.
Also Read This: How to Embed a Link into an Image
Step-by-Step Guide to Edit Docker Image
Editing a Docker image might seem tricky at first, but with a step-by-step approach, it becomes manageable. Here's a straightforward guide to help you through the process:
- Pull the Base Image: Start by pulling the image you want to edit. For example, if you want to modify an Ubuntu image, use the command:
docker pull ubuntu
- Run the Image as a Container: Once you have the image, the next step is to run it as a container. This is where you'll make your changes.
This command starts an interactive shell session within the container.docker run -it ubuntu bash
- Make Changes Inside the Container: Now, you can edit the container by installing software, changing configurations, or modifying files. For example, you could install a package using:
apt-get update && apt-get install
- Commit Changes to a New Image: Once you've made your changes, you need to save the modified container as a new image. Use the following command:
This will create a new Docker image that includes all the changes you've made.docker commit
- Test the New Image: Run the new image to ensure the changes are applied correctly. Use:
If everything works as expected, your new image is ready for use!docker run -it bash
That's it! You've now edited a Docker image and committed the changes. You can further refine the image by repeating this process as needed.
Also Read This: How to Use VectorStock for Valentine’s Day Designs and Promotions
Testing Your Edited Docker Image
Once you've edited your Docker image, it's crucial to test it to ensure everything works as expected. Testing helps catch any issues early and ensures your edited image is functional. Here's how you can do it:
- Run the Image in a Container: The first step is to run the newly created image as a container. This will allow you to see if the changes you made are functional in a live environment. Use the following command:
docker run -it bash
- Verify Installed Software: If you installed new software or tools inside the image, check to make sure they are properly installed and working. For example, if you added a web server, try to start it and access it from your browser.
- Test Configuration Changes: If you modified any configurations (such as environment variables or file paths), make sure they’re correctly applied inside the container. For example, check if database connections are working or if environment variables are properly set.
- Check for Errors: Monitor the container's logs for any errors or issues. You can view the logs by running:
Look for any warning or error messages that might indicate issues with the image.docker logs
- Run Automated Tests (Optional): If you have automated tests for your application, running them inside the container is a great way to validate the functionality of the image. This will give you a more thorough check of how well your changes work.
If everything looks good and works as expected, your edited Docker image is ready for deployment or sharing. However, if you run into issues, you may need to tweak the image or fix any errors before it's ready to go live.
Also Read This: Finding the Pixel Size of an Image
Best Practices for Editing Docker Images
When it comes to editing Docker images, following best practices can save you a lot of time, effort, and confusion. By adhering to some simple guidelines, you can ensure that your images are optimized, maintainable, and efficient. Here are some best practices you should consider:
- Use a Minimal Base Image: Start with a minimal base image, like
alpine
, and only install what you need. This will keep your image small and more secure by reducing its attack surface. - Leverage Dockerfile: Rather than making changes directly in a running container, use a Dockerfile. This allows you to define the steps for building an image in a repeatable and version-controlled manner. Here's an example Dockerfile structure:
FROM ubuntu RUN apt-get update && apt-get install -y python3 COPY . /app CMD ["python3", "/app/app.py"]
- Keep Layers Minimal: Each command in a Dockerfile creates a new layer in the image. Keep your Dockerfile optimized by combining related commands to reduce the number of layers and make the image smaller. For example:
RUN apt-get update && apt-get install -y python3 && apt-get clean
- Use Caching Wisely: Docker caches layers to speed up image builds. However, be mindful of how you structure your Dockerfile. If you frequently change code but not dependencies, put the
COPY
orADD
commands towards the end of the Dockerfile so that Docker can cache earlier layers (like installing dependencies) and only rebuild the necessary parts. - Clean Up After Yourself: Always remove temporary files, caches, or unnecessary dependencies after installation. This will help keep your image lean and avoid bloating. For example, after installing a package, run
apt-get clean
to remove cached package files. - Tag Your Images Clearly: When creating or editing an image, tag it with a meaningful name and version number. This makes it easier to track changes and maintain multiple versions. Use semantic versioning (e.g.,
myapp:1.0
,myapp:1.1
) to organize your images logically.
By following these best practices, you'll ensure that your Docker images remain efficient, easy to maintain, and secure.
Also Read This: How to Merge Videos Together on YouTube
Common Issues and Troubleshooting
Even with the best preparation and practices, issues can arise when editing Docker images. Here’s a look at some of the most common problems and how to troubleshoot them effectively:
- Image Build Failures: If your image fails to build, it’s usually due to incorrect commands in your Dockerfile. Check the Docker build logs to identify the specific line causing the issue. Common issues include incorrect package names, missing dependencies, or permissions issues.
- Missing Dependencies: If the container fails to run due to missing dependencies, check the Dockerfile for the proper installation commands. Sometimes, you may need to install additional packages that were overlooked. Ensure that
apt-get update
is run before installing packages. - Container Not Starting: If your container won’t start, you can check the logs by running:
This will show you any errors or issues preventing the container from running. Common problems include missing environment variables, incorrect port mappings, or application crashes.docker logs
- File Permissions Issues: Permission errors are common, especially when files are copied into the container. Make sure the user running the container has the necessary permissions to access the files. You can change file permissions using the
chmod
command inside the container. - Excessive Image Size: If your Docker image is too large, check for unnecessary files or layers that can be removed. Use the
docker history
command to inspect the image size and find any oversized layers. - Network Issues: If your container can’t connect to external networks or services, make sure the container’s network settings are correctly configured. Ensure that ports are properly exposed and mapped when running the container. You can also use Docker’s
--network
flag to specify the network.
By diagnosing the issue step by step and checking the logs, you can resolve most common Docker image problems quickly and efficiently.
Also Read This: Follow This Easiest Way to Convert iFunny Videos into MP4 Format
FAQ
Here are some frequently asked questions related to editing Docker images that may help clarify some common queries:
- How do I know which Docker image to edit?
It's best to start with a base image that closely matches your needs. For example, if you're working with Python, use an official Python image. If you need a lightweight option, you can choose Alpine-based images. - Can I edit a Docker image without a Dockerfile?
Yes, but it's not recommended. Using a Dockerfile ensures that your changes are repeatable and version-controlled. Without a Dockerfile, you'd need to manually commit changes from a running container, which is less efficient and harder to manage. - How do I push an edited Docker image to Docker Hub?
After editing and committing your image, use the following command to push it to Docker Hub:
Make sure you're logged into your Docker Hub account first usingdocker push /:
docker login
. - What should I do if my container doesn't start after editing the image?
If your container isn't starting, check the logs for any error messages. Ensure all dependencies are correctly installed and that you’ve set the right environment variables. You can view logs withdocker logs
. - How do I roll back to a previous Docker image version?
If you’ve created multiple versions of an image, you can revert to a previous version by using the image tag associated with that version. For example:docker run -it :
If you have other questions or encounter any issues not addressed here, feel free to reach out to the Docker community or consult the official Docker documentation for additional support.
Conclusion
Editing Docker images is an essential skill for developers looking to optimize their workflows, improve application deployment, and ensure that their containers are running smoothly. By understanding the process and following best practices, you can create efficient and secure Docker images tailored to your project’s needs. Remember to keep your images lightweight, use Dockerfiles to automate changes, and regularly test your images to ensure functionality. Docker’s flexibility allows for quick changes and scalability, so don’t hesitate to dive in and start experimenting. With the right tools and knowledge, you can master Docker image editing and take full advantage of the power Docker offers.