In web design, images are essential to make the user interface engaging and visually appealing. React, a popular JavaScript library for building user interfaces, offers various ways to add images to your web applications. Whether it's for displaying product images, showcasing portfolio pieces, or creating a dynamic experience, images help enhance user engagement. In this section, we'll explore why adding images in React is crucial and how to do it effectively.
Understanding the Basics of React Image Handling
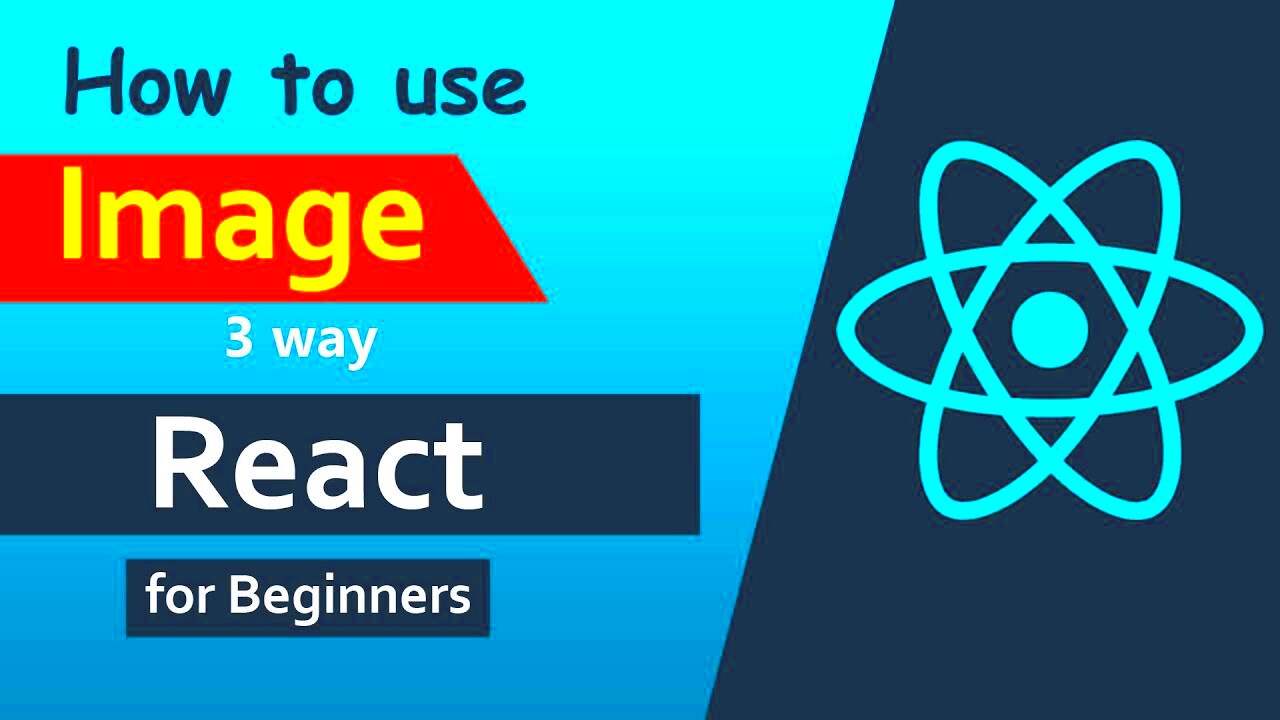
When working with React, managing images is a bit different than in traditional web development due to React's component-based structure. React allows you to import and manage images efficiently within your components. Here’s a breakdown of key concepts to help you understand React’s image handling:
- Static Images: Images stored locally in your project can be imported and used within your components.
- Dynamic Images: React allows you to load images dynamically, depending on the user’s interaction or state changes.
- Public Folder: Images can also be stored in the public folder and referenced via a relative URL.
React’s flexibility in handling images ensures that you can add them in ways that make your application more interactive and responsive to user needs. The key is understanding how React’s image management works and how to integrate it seamlessly into your app.
Different Methods to Add Images in React
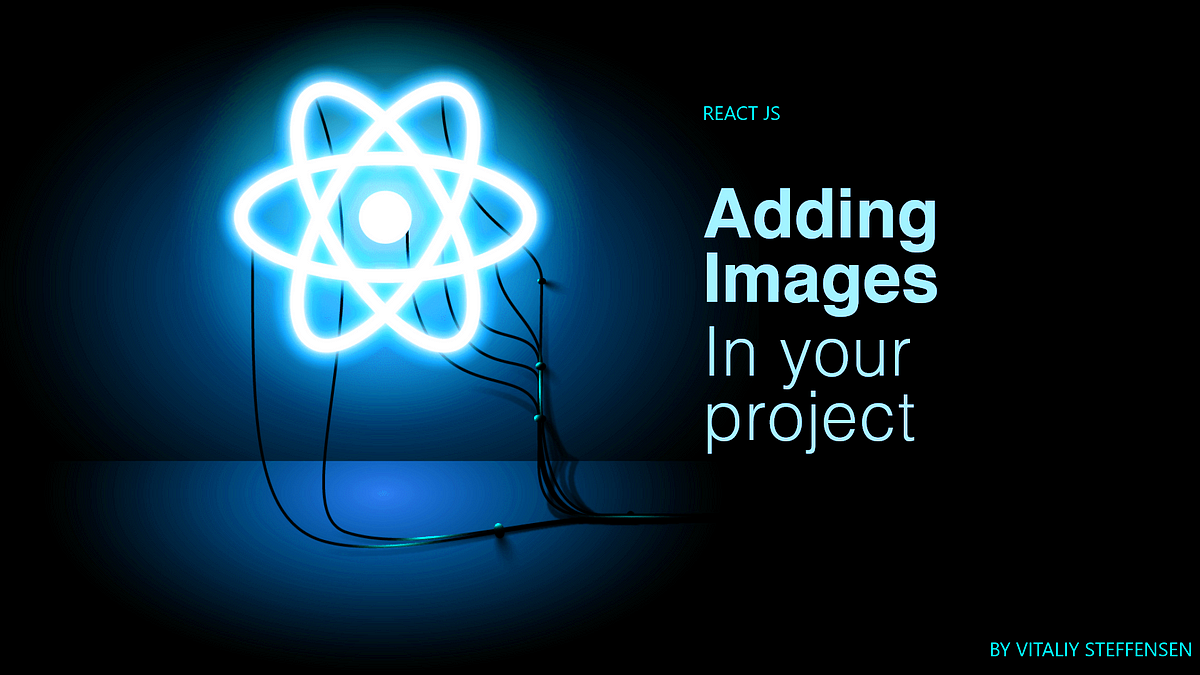
There are several ways to add images in React, depending on your project structure and how you want the images to be displayed. Below are some of the most common methods:
1. Importing Images
One of the simplest ways to add images is by importing them directly into your React components. This method ensures that images are bundled and optimized by Webpack when building the application.
import MyImage from './assets/myimage.jpg';
Then, you can use it like this:
<img src={MyImage} alt="Description" />
2. Using Images from the Public Folder
If you want to use images that are not bundled with your app, you can place them in the public folder and reference them directly by their relative path.
<img src="/images/myimage.jpg" alt="Description" />
This method is great for static assets that don’t need to be imported and are directly accessible by users via a URL.
3. Dynamically Loading Images
If you want to display images dynamically based on state or props, React allows you to reference images conditionally. For example, you can load an image based on the data fetched from an API.
<img src={this.state.imageUrl} alt="Dynamic Image" />
This method is helpful when the images are part of a dynamic list or when they change based on user input.
4. Using Image URLs
Another method is using external image URLs from a CDN or image hosting service. This is often useful for loading images that are not part of your project but are hosted online.
<img src="https://example.com/myimage.jpg" alt="External Image" />
These are some of the most common ways to add images in React. Each method has its use case depending on your project needs. Choosing the right approach can improve your app’s performance, loading speed, and overall user experience.
How to Use Images in React Components
Using images in React components is straightforward, but it’s important to do it in a way that maintains clean code and good performance. In React, you can add images by either importing them or referencing them through a URL. The goal is to ensure images load properly and fit well into the design. Let’s go over how to effectively use images in your React components.
1. Importing Images into Components
The simplest way to use images in React is to import them into your component file. This allows Webpack to bundle them with your project, which ensures better performance and reduces issues with paths.
import Logo from './assets/logo.png';
Then you can use the image like this:
<img src={Logo} alt="Logo" />
2. Using Images Stored in the Public Folder
If you prefer not to import images, you can store them in the public folder. The public folder is accessible throughout the project, and images stored there can be used by specifying their relative path.
<img src="/images/my-image.jpg" alt="My Image" />
This is ideal for static images that don’t need to be part of the build process.
3. Dynamically Displaying Images Based on State or Props
Another common use case is dynamically changing images based on state or props. This is useful when you want to load different images based on user interactions, such as clicking a button or selecting an item.
<img src={this.state.imageUrl} alt="Dynamic Image" />
These methods make it easy to use images in React components while ensuring flexibility and clean code.
Optimizing Images for Performance in React
Images can significantly affect the performance of your React app, especially when they are large in size or not optimized. Slow-loading images can negatively impact user experience, so it’s crucial to optimize them for faster load times. Let’s explore some ways to improve image performance in React.
1. Compressing Images
One of the first steps in optimizing images is compressing them without sacrificing quality. Tools like ImageOptim, TinyPNG, or Squoosh can help reduce file size.
- Lossy Compression: Reduces the file size by slightly degrading the image quality (but often imperceptible to the user).
- Lossless Compression: Maintains the original quality but still reduces the size.
2. Using Modern Image Formats
Formats like WebP provide better compression than traditional formats like JPEG and PNG, making them a good choice for improving load times. WebP images can often be 25-35% smaller than PNGs and JPEGs.
3. Lazy Loading Images
Lazy loading ensures that images are only loaded when they are about to be displayed on the user’s screen. This helps reduce the initial loading time and saves bandwidth. You can implement lazy loading with the `loading="lazy"` attribute:
<img src="myimage.jpg" alt="Lazy Loaded" loading="lazy" />
4. Image Sprites
If your app uses several small images, consider combining them into a single image sprite. This reduces the number of HTTP requests and can improve performance.
Optimizing images is an important step in improving the speed and overall user experience of your React app.
Handling Responsive Images in React
In today’s world, websites must be mobile-friendly and adapt to different screen sizes. Handling responsive images in React ensures that your images look great on any device. Let’s take a look at how you can implement responsive images in your React app.
1. Using the `` Element
The `` element is a powerful tool for displaying different images based on the device’s screen size. This approach allows you to serve different image sizes for different devices, ensuring images are optimized for performance.
<picture>
<source srcSet="small-image.jpg" media="(max-width: 600px)" />
<source srcSet="large-image.jpg" media="(min-width: 601px)" />
<img src="default-image.jpg" alt="Responsive Image" />
</picture>
This method allows React to automatically choose the best image for the user’s screen size.
2. Using CSS for Responsiveness
In some cases, you can use CSS to make images responsive. The following CSS properties ensure that images scale proportionally to the container’s size:
img {
width: 100%;
height: auto;
}
This method works best for images that don’t need to change based on screen size but should adjust to fit the container.
3. The `srcSet` Attribute for Dynamic Resizing
The `srcSet` attribute allows you to define multiple sizes of an image, and the browser will choose the appropriate one based on the screen resolution and size. Here’s an example:
<img srcSet="image-small.jpg 600w, image-medium.jpg 1200w, image-large.jpg 2000w" alt="Responsive Image" />
This ensures that high-resolution devices (like Retina displays) get higher-quality images, while smaller devices load lighter images.
4. Media Queries for Responsive Design
Media queries are essential for making images responsive to different screen sizes. You can use them in your CSS to adjust image properties based on the width of the viewport:
@media (max-width: 768px) {
img {
width: 100%;
height: auto;
}
}
By using these techniques, you can ensure that your images look sharp and load quickly across a wide variety of devices.
Common Issues and How to Fix Them When Using Images in React
When working with images in React, there are several common issues developers encounter. These problems can range from broken image links to performance issues. Understanding how to diagnose and fix these issues can save you a lot of time and ensure your app looks and functions as expected. Let’s explore some of the most common problems and their solutions.
1. Broken Image Links
One of the most common issues is broken image links, which happen when an image’s source path is incorrect. This can be caused by typos, incorrect file paths, or missing images in your project.
- Solution: Double-check the image path and ensure the image is located in the correct folder. If you're importing images, confirm the import statement is correct.
- Solution: For public folder images, ensure the path is relative to the public directory (e.g.,
/images/myimage.jpg
).
2. Slow-Loading Images
Images can significantly slow down your app if they’re not optimized. Large image files increase load times and decrease performance.
- Solution: Use image compression tools like TinyPNG or ImageOptim to reduce file sizes without compromising quality.
- Solution: Consider using modern image formats like WebP, which offer smaller file sizes with similar or better quality than PNG or JPEG.
3. Images Not Displaying in Different Screen Sizes
Responsive design issues can occur when images do not adjust properly to different screen sizes or devices, causing them to look distorted or cropped.
- Solution: Use the
width: 100%
andheight: auto
CSS properties to ensure images scale correctly. - Solution: Implement the `` element or `srcSet` for more control over different image sizes for different devices.
4. Caching Issues
Sometimes, changes to images may not be reflected immediately due to browser caching, where the browser stores old versions of images.
- Solution: Clear the cache or use versioning by adding query strings to the image URLs (e.g.,
image.jpg?v=1
) to force the browser to load the new image.
Best Practices for Image Usage in React
Incorporating images into your React projects is essential for engaging design, but it’s also important to follow best practices to optimize performance, maintainability, and accessibility. Let’s look at some key best practices for image usage in React.
1. Optimize Image Size and Format
Large images can slow down your application, so it’s important to optimize both the file size and format. Consider the following best practices:
- Compress images: Use tools to reduce file size without sacrificing too much quality.
- Choose the right format: Use modern formats like WebP for smaller file sizes. For vector images, SVG is an excellent choice.
2. Use Lazy Loading
Lazy loading helps improve performance by loading images only when they are about to be displayed on the user’s screen. This technique reduces initial page load time.
- Solution: Add the
loading="lazy"
attribute to your images to enable lazy loading.
3. Use Responsive Images
To ensure your images look great on all devices, use responsive images. You can implement responsive images by using the srcSet
attribute, CSS techniques, or the `` element.
- Solution: Define multiple sizes of images using `srcSet` for varying screen resolutions.
- Solution: Use media queries to adjust image sizes based on screen size.
4. Accessibility Considerations
For better accessibility, make sure that every image has an alt
attribute. This helps users who rely on screen readers and also improves SEO.
- Solution: Always include a descriptive
alt
text for images, especially if they are functional or decorative.
5. Keep Your Code Clean
Ensure your image imports and references are well-organized, especially in larger React projects. Keeping things clean helps maintain the scalability of your codebase.
- Solution: Group image imports and references logically to improve maintainability.
FAQ
Here are some common questions developers have when working with images in React.
1. How can I display images in React?
You can display images in React by either importing them into your components or using URLs to reference images stored in the public folder or externally hosted images. The most common methods include using import
statements or src
attributes with paths to image files.
2. What is lazy loading, and why should I use it?
Lazy loading is a technique that delays the loading of images until they are about to be displayed on the user’s screen. This reduces the initial load time and improves performance, especially on image-heavy websites.
3. What is the best image format for React?
Modern formats like WebP provide excellent compression and quality, making them a great choice for React apps. For vector images, consider using SVG, which scales well and often has smaller file sizes than other formats.
4. How can I make images responsive in React?
You can make images responsive in React by using CSS techniques such as width: 100%
and height: auto
, or you can use the `` element or the `srcSet` attribute to serve different image sizes for different screen resolutions and devices.
5. How do I handle broken image links in React?
If you encounter broken image links, ensure the file path is correct. Double-check whether the image is in the right folder, and for images in the public folder, confirm the relative path. If the image is imported, verify the import statement is accurate.
Conclusion
In conclusion, adding images in React is an essential part of creating visually appealing and interactive web applications. By understanding how to effectively handle images through various methods like importing, referencing URLs, and dynamically loading them, you can ensure that your React projects are both user-friendly and performant. It's crucial to also focus on optimizing images for speed, using responsive techniques for different devices, and following best practices for accessibility and code cleanliness. By addressing common issues and adhering to these strategies, you can provide an excellent user experience while keeping your web applications running smoothly.