Adding images dynamically using JavaScript can elevate your web projects by enhancing interactivity and user experience. Whether you want to display images based on user actions or pull content dynamically from an API, JavaScript makes it seamless. In this post, we’ll cover the basics of how to insert images into your web pages using JavaScript, making your sites more engaging and interactive!
Understanding the DOM and Image Elements
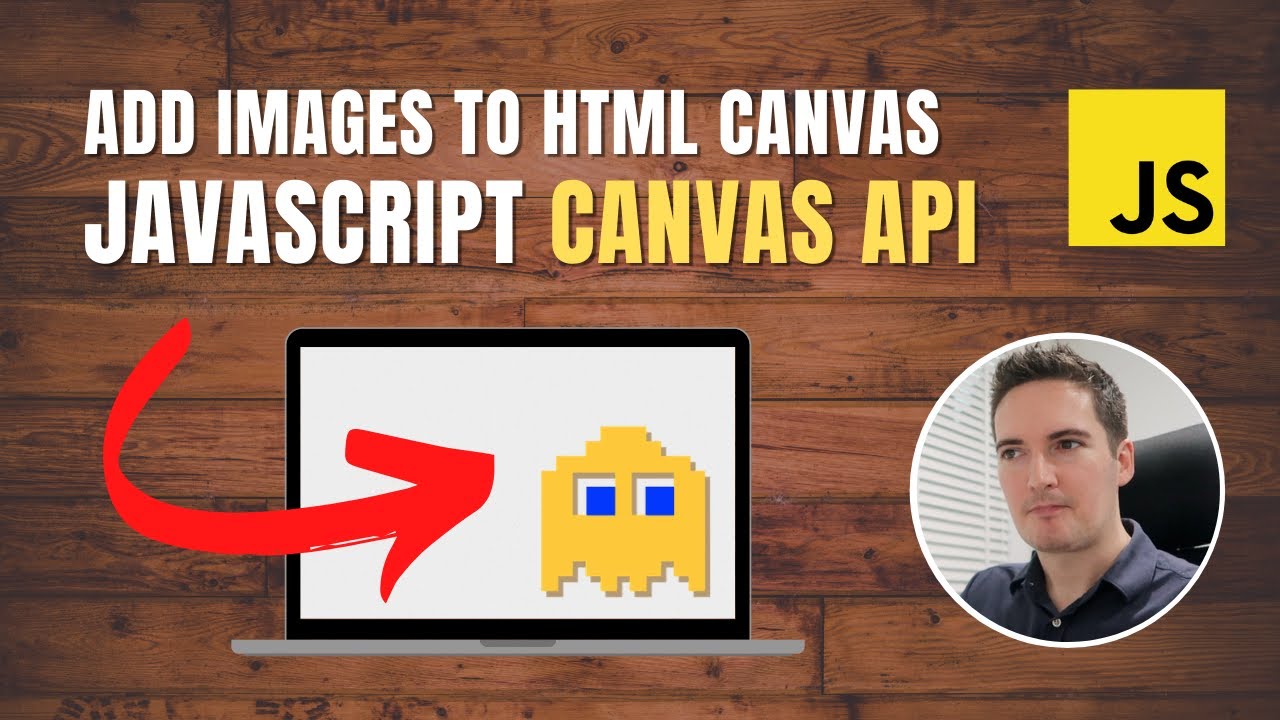
Before diving into adding images, it’s crucial to understand the Document Object Model (DOM). The DOM is a representation of the structure of your HTML document. It allows you to interact with and manipulate content, structure, and styles through JavaScript. Images, being integral to web content, are represented as <img>
elements within this structure.
Here’s a quick breakdown of what you need to know:
- DOM Structure: The DOM represents the HTML document as a tree of objects, which can be manipulated with JavaScript. Each element, such as
<div>
,<h1>
, or<img>
, is a node in this tree. - Image Element: The
<img>
element is the HTML tag used to embed images in a document. It consists of several attributes, including src (source), alt (alternative text), and more. - JavaScript Interaction: You can use JavaScript to manipulate these image elements, such as creating new images, changing their attributes, or removing them from the DOM.
To add an image using JavaScript, you typically follow these steps:
- Create an Image Element: Use the
document.createElement()
method to create a new image element. - Set Attributes: Assign the
src
andalt
attributes to the newly created image element. - Append to DOM: Finally, append the new image element to the desired location in the DOM using methods like
appendChild()
.
Here’s a simple code snippet that illustrates this process:
function addImage() {
// Create a new img element
var img = document.createElement('img');
// Set the source and alt attributes
img.src = 'https://example.com/image.jpg';
img.alt = 'An example image';
// Append the image to a div with an id of "imageContainer"
document.getElementById('imageContainer').appendChild(img);
}
In this example, when the addImage()
function is called, it creates a new image element and attaches it to the DOM where you specify. This approach not only simplifies adding images but also allows for dynamic updates based on user interactions or other events. So, the next time you think about incorporating images into your web applications, remember: the DOM and JavaScript together make it a breeze!
Also Read This: Printing a Large Image Across Multiple Pages
3. Using the createElement Method to Add Images
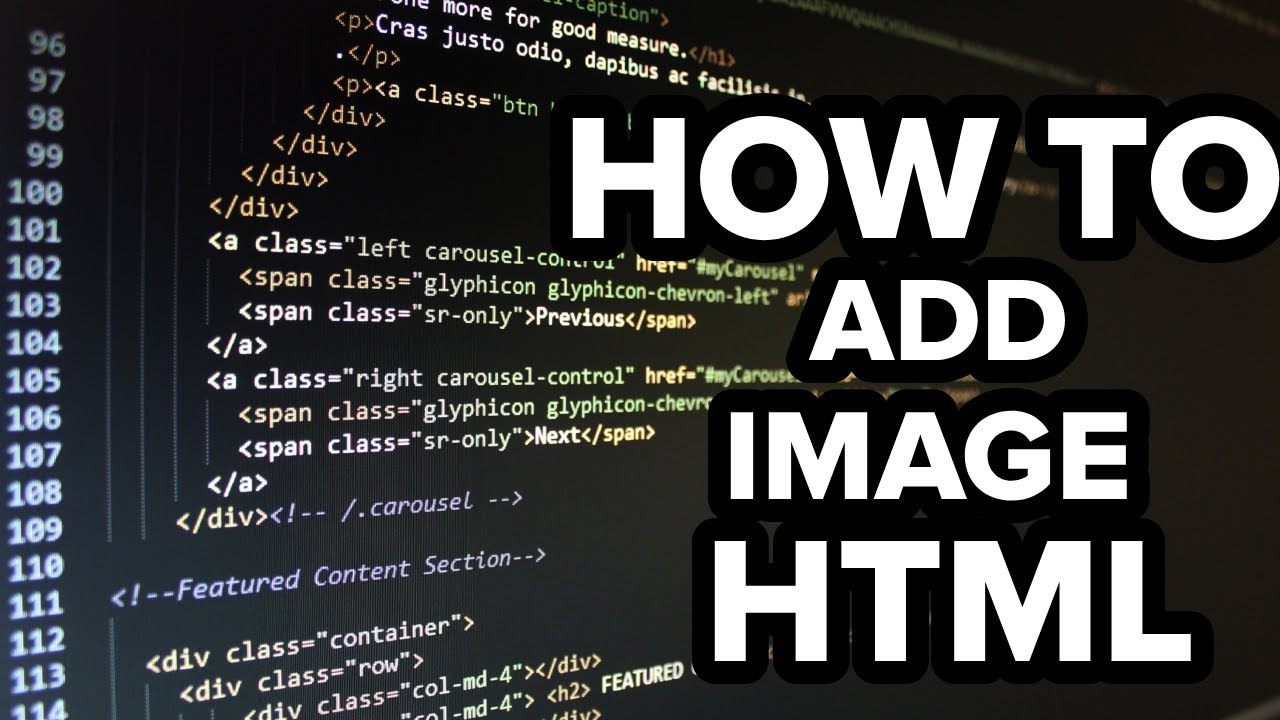
So, you’re eager to spruce up your webpage with some eye-catching images, and you want to do it dynamically using JavaScript. One of the cleanest ways to achieve this is by using the createElement
method. Let’s break it down step by step!
First off, the createElement
method is a part of the Document Object Model (DOM) in JavaScript, which allows you to create new HTML elements on the fly. For our purpose, we’ll specifically create an img
element.
Here’s how you can go about it:
const img = document.createElement('img');
With this line, you’ve just created an img
element, but it’s not displayed anywhere yet. It’s like having the picture ready but not hanging it on the wall! Next, you’ll want to define where you’re going to put this image.
Let’s say you have a <div>
in your HTML with the ID of image-container
. You can append your newly created image to this div like so:
document.getElementById('image-container').appendChild(img);
Now, your image is part of the DOM, but it’s still missing some key details. You’ll want to set its source and possibly some other attributes, which brings us to the next section!
Here’s a simple example of how your final code might look:
const img = document.createElement('img');
img.src = 'path/to/your/image.jpg';
img.alt = 'A descriptive alt text for the image';
document.getElementById('image-container').appendChild(img);
That's it! You’ve dynamically created an image element and added it to your webpage. This method gives you flexibility and control over how and when images are added, making it a powerful tool in your JavaScript arsenal.
Also Read This: Making Money through Adobe Stock or Shutterstock
4. Setting Image Attributes in JavaScript
Now that you've created your image, let’s dive into setting its attributes. Attributes are like little details that give context to your image, such as its source, dimensions, and even alternative text for accessibility. It's essential to keep these in mind when adding images using JavaScript!
Here are some of the most common attributes you'll want to set for your images:
- src: This is the most crucial attribute that specifies the path to your image.
- alt: This attribute provides alternative text for the image, which is essential for screen readers and will display if the image fails to load.
- width: Set the width of your image. You can specify it in pixels (px) or as a percentage (%).
- height: Similar to width, this sets the height of your image.
- title: This gives additional information about the image when the user hovers over it.
Let's look at how to apply these attributes using JavaScript:
const img = document.createElement('img');
img.src = 'https://example.com/path/to/image.jpg';
img.alt = 'A beautiful scenery';
img.width = 500; // Width in pixels
img.height = 300; // Height in pixels
img.title = 'Click for more information';
document.getElementById('image-container').appendChild(img);
By setting these attributes, you're not just adding an image; you're also enhancing the user experience and catering to web accessibility standards.
So there you have it! Using the createElement
method provides you with a powerful way to dynamically insert images into your webpage, while setting attributes ensures that those images are functional and accessible. Happy coding!
Also Read This: how to split a large image on cricut
5. Appending the Image to the Document
Now that you have your image element created and properly configured, it's time to add it to the document. This process is straightforward, and with just a few lines of code, you can see your image appear on the web page!
Here's how you can do it:
- First, ensure you have a reference to the parent element where you want to insert the image. This could be a
div
,section
, or any other container. - Next, use the
appendChild()
method, which allows you to add the image element to your chosen parent element.
Here’s a simple example to illustrate:
// 1. Create the image element
const img = document.createElement('img');
// 2. Set the source attribute
img.src = 'https://example.com/image.jpg';
// 3. Set other attributes (optional)
img.alt = 'A lovely scenery';
img.style.width = '300px';
img.style.height = 'auto';
// 4. Get the parent element
const parentElement = document.getElementById('image-container');
// 5. Append the image to the document
parentElement.appendChild(img);
In this code:
- We create a new
img
element and set its source. - We optionally set an
alt
text and style attributes for width and height. - We then retrieve the parent element using its ID and append the image to it.
When you run this code, you should see your image appear in the specified container on your web page. If the image isn’t showing up, check the following:
- Make sure the image URL is correct
- Verify the parent element ID matches the one used in your JavaScript
- Check for any JavaScript errors in the console
And just like that, you’ve successfully added an image to your web page using JavaScript!
Also Read This: How to Record Songs from YouTube
6. Loading Images Dynamically Using JavaScript
In some cases, you might want to load images dynamically based on user interaction or other events. This can make your application feel more responsive and interactive. Let’s explore how you can achieve that.
For instance, imagine a scenario where a user clicks a button to load a new image. You can handle this easily with JavaScript. Here’s how:
// HTML button
// JavaScript code
document.getElementById('loadImageBtn').addEventListener('click', function() {
const img = document.createElement('img');
img.src = 'https://example.com/new-image.jpg';
img.alt = 'A new scenery';
img.style.width = '300px';
img.style.height = 'auto';
const parentElement = document.getElementById('image-container');
parentElement.innerHTML = ''; // Optional: Clear the existing image
parentElement.appendChild(img);
});
In this example:
- We’ve set up a button with an ID of
loadImageBtn
. - We attach an event listener to this button that triggers when it’s clicked.
- When the button is clicked, a new image element is created and appended to the container.
- Setting
parentElement.innerHTML = ''
allows us to clear the previous image, but you can omit this line if you want to keep the old images on display.
This method is particularly useful for galleries, sliders, or any dynamic content where images might change based on user actions. Just remember to keep accessibility in mind by providing meaningful alt
text for each image!
Loading images dynamically can greatly enhance user experience and make your web applications feel modern and sleek. So go ahead and experiment with different scenarios where loading images on demand can make your site more appealing.
Also Read This: Unprivate Your Projects on Behance for Public Viewing
7. Handling Errors When Adding Images
When you're working with images in JavaScript, it’s essential to anticipate the possibility of errors. After all, what happens if an image fails to load? That's where error handling comes into play, and it can make your web application more robust and user-friendly. Let’s look at how you can effectively manage errors when adding images.
First things first, utilizing the onerror
event is a great way to catch image-loading issues. This method allows you to execute a function if an image fails to load, giving you the flexibility to respond appropriately.
Here’s a simple example:
const img = new Image();
img.src = 'path/to/your/image.jpg';
img.onerror = function() {
console.error('Image failed to load.');
document.body.innerHTML += 'Oops! The image could not be loaded.
';
};
In the above example, if the image specified in the src
fails to load, the onerror
function will execute, logging an error message in the console and displaying a notification to the user.
Another tip is to implement a fallback mechanism. This means providing an alternative image if the primary one cannot be loaded. Here’s how to do that:
const img = new Image();
img.src = 'path/to/your/image.jpg';
img.onerror = function() {
this.onerror = null; // Prevent infinite loop
this.src = 'path/to/your/fallback-image.jpg';
};
In this case, if the first image fails, the code will try to load a fallback image instead.
Lastly, never forget to consider how errors might affect your users. A broken image could lead to a poor experience, so having a visual cue that something went wrong is essential. Use placeholder images, descriptive error messages, or even a search option if the image is essential for conveying information.
Also Read This: How Much YouTube Pays for a 1-Hour Video Revenue Insights
8. Best Practices for Using Images in Web Development
Using images in web development is more than just about aesthetics; it's about performance, accessibility, and ensuring a seamless experience for your users. Here are some best practices to keep in mind when incorporating images into your projects.
- Optimize Image Sizes: Always use properly sized images for your webpages. Large images can slow down your site, affecting both performance and user experience. Use tools like TinyPNG or ImageCompressor to reduce the file size without significant quality loss.
- Use the Right Format: Different image formats serve different purposes. Use JPEG for photographs, PNG for images that require transparency, and SVG for vector graphics. Choosing the right format can significantly impact loading speed.
- Add Alt Text: Always include descriptive alt text for your images. This not only assists with accessibility for visually impaired users but also helps with SEO. Screen readers use the alt attribute to describe images.
- Lazy Loading Images: To improve webpage load time, consider implementing lazy loading for images. This technique delays loading of images until they are in the viewport, helping to boost performance.
- Responsive Images: Use responsive image techniques like the
<picture>
element orsrcset
attribute. This allows you to serve different images based on the user’s device size and resolution, ensuring optimal viewing experiences.
In addition to the above practices, always keep user experience at the forefront. Take the time to test how images render on different devices and browsers. This not only helps in avoiding broken images but also ensures your site is visually consistent across platforms. Remember, your web application’s success is deeply tied to how it visually communicates with your users, and images are a fundamental part of that narrative!
Conclusion and Further Resources
In summary, adding images dynamically to your web pages using JavaScript can greatly enhance user interaction and experience. By mastering the methods discussed above, you can create more engaging and visually appealing content. Whether you choose to manipulate the DOM directly or use frameworks, understanding how to incorporate images effectively will elevate your web development skills.
Here are some useful resources to further enhance your knowledge:
- MDN Web Docs: createElement() - An in-depth guide on creating HTML elements.
- W3Schools: HTML DOM Elements - A tutorial on how to access and manipulate HTML elements with JavaScript.
- JavaScript.info - A comprehensive guide to JavaScript, covering everything from the basics to advanced topics.
- CSS-Tricks: Using the HTML5 Image Element - Insights on effectively using images in HTML5.
Utilize these resources to deepen your understanding and improve your coding techniques. Happy coding!