Embedding images in a canvas element is an essential technique in web design and development. It allows you to display images within interactive graphics or visual content that can be manipulated with JavaScript. A canvas is a versatile element in HTML5 that provides a space for dynamic graphics, such as drawings, animations, and images. When you embed an image in a canvas, you can control how the image appears, adjust its size, and even apply effects. Understanding how to embed images properly ensures that your content looks great and functions as intended.
Why You Should Embed Images in Canvas
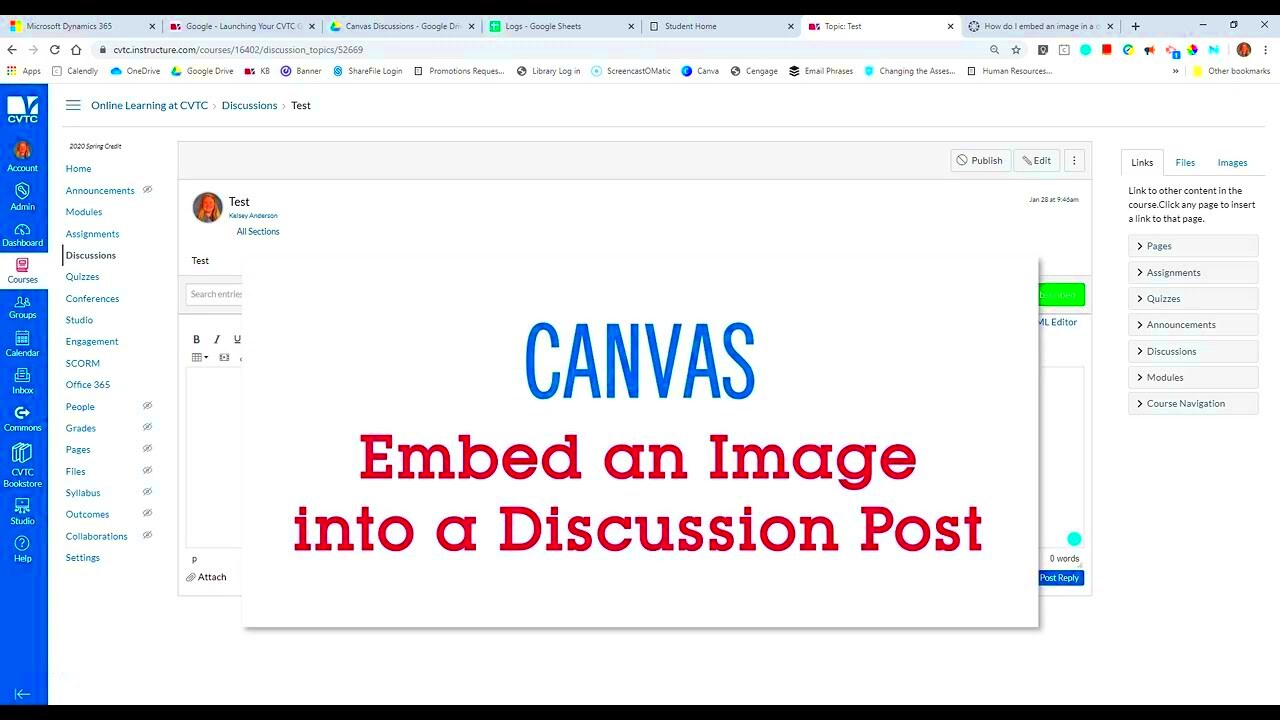
Embedding images in canvas is a powerful way to enhance your web projects. Here’s why it’s worth learning:
- Customizability: You have full control over the image’s position, size, and style within the canvas.
- Interactivity: Canvas allows you to interact with images using JavaScript, which is useful for games, interactive graphics, and data visualization.
- Efficient Rendering: With canvas, you can render complex graphics, such as charts or custom effects, quickly and efficiently.
- Flexibility: You can manipulate images, apply filters, or animate them within the canvas element, giving you greater design flexibility.
Embedding images in canvas is an essential skill for creating dynamic and interactive user experiences. Whether for an online game, interactive graphic, or custom image editing tool, the canvas element is a valuable resource in modern web development.
Also Read This: Exciting Job Openings in Digital Image Processing Across the USA
How to Embed Images in Canvas Using HTML
Embedding an image in canvas is relatively simple, and it involves using both HTML and JavaScript. Here’s a step-by-step guide:
- Step 1: Create the canvas element in HTML.
<canvas id="myCanvas" width="500" height="500"></canvas>
In this step, you define the size of the canvas by setting the width and height attributes. Make sure to give it an ID so you can easily reference it later.
- Step 2: Use JavaScript to load the image and draw it onto the canvas.
let canvas = document.getElementById('myCanvas');
let ctx = canvas.getContext('2d');
let img = new Image();
img.src = 'path-to-your-image.jpg';
img.onload = function() {
ctx.drawImage(img, 0, 0);
};
Here, we use the getContext method to access the canvas’s 2D context, which allows us to draw on the canvas. The drawImage
method is used to place the image at the specified position (in this case, at the top-left corner of the canvas).
- Step 3: Ensure the image is loaded before drawing it on the canvas.
Using the onload
event ensures that the image is fully loaded before it’s drawn, preventing any errors from occurring if the image hasn’t been loaded in time.
That’s all it takes to embed an image in a canvas using HTML and JavaScript! You can now further manipulate the image by adjusting its size, applying filters, or creating animations.
Also Read This: Tips for Getting Approved as a Contributor to Getty Images
Embedding Images in Canvas with JavaScript
Embedding images in a canvas with JavaScript gives you the power to dynamically manipulate images, making them part of interactive designs and graphics. Using JavaScript, you can not only draw images onto the canvas but also animate, resize, or apply effects to them. The key to embedding an image into a canvas is using the drawImage
method in combination with the canvas’s 2D context. This method allows you to specify the image’s position, size, and even crop it if needed.
Here’s how to embed an image using JavaScript:
- Define the canvas and get its context: First, select the canvas element and access its 2D context with
getContext('2d')
. This will allow you to draw on the canvas. - Create an Image object: You need to create a new
Image()
object, set its source (the path to your image), and use theonload
event to ensure the image loads before drawing. - Draw the image: Once the image is loaded, you can use
drawImage()
to place it on the canvas.
Example code:
let canvas = document.getElementById('myCanvas');
let ctx = canvas.getContext('2d');
let img = new Image();
img.src = 'path-to-your-image.jpg';
img.onload = function() {
ctx.drawImage(img, 50, 50, 200, 200); // Draw image at position (50, 50) with size 200x200
};
By embedding images with JavaScript, you unlock the ability to create rich, interactive graphics that can respond to user actions, animations, and more.
Also Read This: Easy Method to Record Telegram Video Call With Audio
Adjusting Image Size After Embedding
One of the benefits of embedding images in canvas is that you can easily adjust the image's size after it’s been placed. Whether you want to resize the image to fit a specific area or apply a scaling effect, canvas gives you the flexibility to make these adjustments on the fly.
To adjust the image size in canvas, use the drawImage()
method. It accepts four parameters: the image, the X and Y coordinates to draw the image, and the width and height to scale the image to.
- Width and Height: Specify the width and height of the image you want to display on the canvas.
- Aspect Ratio: Be mindful of the image's aspect ratio to prevent it from appearing stretched or distorted. If you want to maintain the original proportions, calculate the width and height based on the aspect ratio.
Example:
let img = new Image();
img.src = 'image.jpg';
img.onload = function() {
let scaleWidth = 300;
let scaleHeight = img.height * (scaleWidth / img.width);
ctx.drawImage(img, 0, 0, scaleWidth, scaleHeight);
};
This example scales the image's width to 300 pixels while maintaining the original aspect ratio. You can also use other methods to crop the image, zoom in, or create custom resizing effects, depending on your project needs.
Also Read This: How to Crop an Image in Google Sheets
Common Issues When Embedding Images in Canvas
While embedding images in canvas is relatively simple, there are a few common issues that developers often face. Understanding and resolving these problems will help ensure a smoother experience when working with canvas images.
- Image not loading: If the image doesn’t appear on the canvas, it might not have finished loading before the
drawImage()
method is called. Always use theonload
event to make sure the image is ready to be drawn. - Cross-origin issues: When trying to load images from a different domain, you might run into cross-origin security restrictions. To solve this, you can either host the image on the same domain or use a CORS-enabled image.
- Image distortion: When resizing images, especially when altering the aspect ratio, they can appear stretched or distorted. To avoid this, make sure to adjust the image’s width and height proportionally, or set one dimension (height or width) and calculate the other.
- Canvas dimensions: The size of the canvas might be too small or too large for the image. If the canvas is too small, your image might be cropped or not visible. Make sure the canvas size matches the image or adjust it accordingly.
- Performance issues: Embedding and manipulating large images in the canvas can cause performance problems, especially with animations or frequent redraws. Consider optimizing the image or using smaller resolutions to improve performance.
By being aware of these common issues and implementing the right solutions, you can ensure smooth image embedding in your canvas projects. Keep testing and refining your code to ensure that your images load correctly and appear as expected.
Also Read This: How to Determine the Resolution of an Image Quickly
Best Practices for Embedding Images in Canvas
Embedding images in a canvas is not just about placing an image on the screen. To get the best results, it’s important to follow certain best practices. By doing so, you ensure that your images appear correctly, load quickly, and don’t negatively affect the user experience. Here are a few tips to keep in mind:
- Optimize Image Size: Before embedding images in the canvas, make sure the image is optimized for the web. Large image files can slow down loading times, so compress the image without sacrificing too much quality.
- Use Proper Image Formats: Choose the right image format based on your needs. For example, use
JPEG
for photos,PNG
for transparent images, andSVG
for scalable graphics. Each format has its strengths and weaknesses. - Handle Image Loading Efficiently: Always make sure that the image is fully loaded before you attempt to draw it on the canvas. Use the
onload
event to ensure that the image is ready, and consider showing a loading animation if needed. - Maintain Aspect Ratio: When resizing images in the canvas, preserve the aspect ratio to avoid distortion. If you need to adjust the size, calculate the appropriate width or height based on the original proportions.
- Consider Canvas Size: Ensure that the canvas dimensions match the image size or are adjusted to fit it properly. This prevents images from being cropped or stretched unexpectedly.
- Handle Cross-Origin Issues: If you're using images from different domains, make sure they are hosted with the correct CORS settings to avoid security issues when drawing them on the canvas.
By following these best practices, you’ll ensure that your images are not only embedded correctly but also optimized for the best performance and user experience.
Also Read This: How to Do Makeup at Home Step by Step with Easy Tips for Beginners
FAQ
Here are some frequently asked questions about embedding images in canvas:
- Q: Can I animate an image inside a canvas?
A: Yes, you can animate images inside a canvas. You can use JavaScript and therequestAnimationFrame
method to create smooth animations, such as moving, rotating, or resizing images. - Q: How can I draw an image at a specific position?
A: To draw an image at a specific location on the canvas, use thectx.drawImage(image, x, y)
method, wherex
andy
are the coordinates on the canvas where you want the image to appear. - Q: Can I use transparent images in canvas?
A: Yes, you can use transparent PNG images in the canvas. When you draw the image, the transparent areas will remain transparent. - Q: What happens if the canvas size is smaller than the image size?
A: If the canvas is smaller than the image, the image may get cropped or not display properly. To avoid this, adjust the canvas size or scale the image to fit. - Q: Can I apply filters to images in canvas?
A: Yes, you can apply various filters to images in canvas, such as grayscale, sepia, blur, etc. Use thectx.filter
property to apply these effects before drawing the image.
Conclusion
Embedding images in canvas is a great way to create interactive and dynamic content for your website or application. By using HTML5 and JavaScript together, you can manipulate images, create animations, and offer a more engaging experience to your users. However, it’s essential to follow best practices to ensure that your images are optimized, load quickly, and display correctly. Remember to consider issues like image size, aspect ratio, and cross-origin restrictions when working with images in canvas.
With the knowledge you’ve gained, you can start embedding images in your canvas projects and take your web designs to the next level. Whether it’s for an interactive graphic, a game, or a custom photo editor, the canvas element provides the flexibility to bring your ideas to life in exciting ways.