When it comes to creating a dynamic website, adding images using JavaScript is a fantastic way to make your content more interactive and engaging. Rather than embedding images statically in HTML, JavaScript allows you to add them dynamically, responding to user actions or events on the page. This method provides flexibility in how images are loaded and displayed, enhancing both functionality and user experience.
By using JavaScript, you can modify or update images in real-time without reloading the entire page. This is especially useful for creating interactive galleries, changing visuals based on user preferences, or even implementing features like image sliders. In this guide, we’ll walk through the process of adding images dynamically and explain how you can leverage JavaScript to make your website content more dynamic.
Why You Should Use JavaScript for Dynamic Images
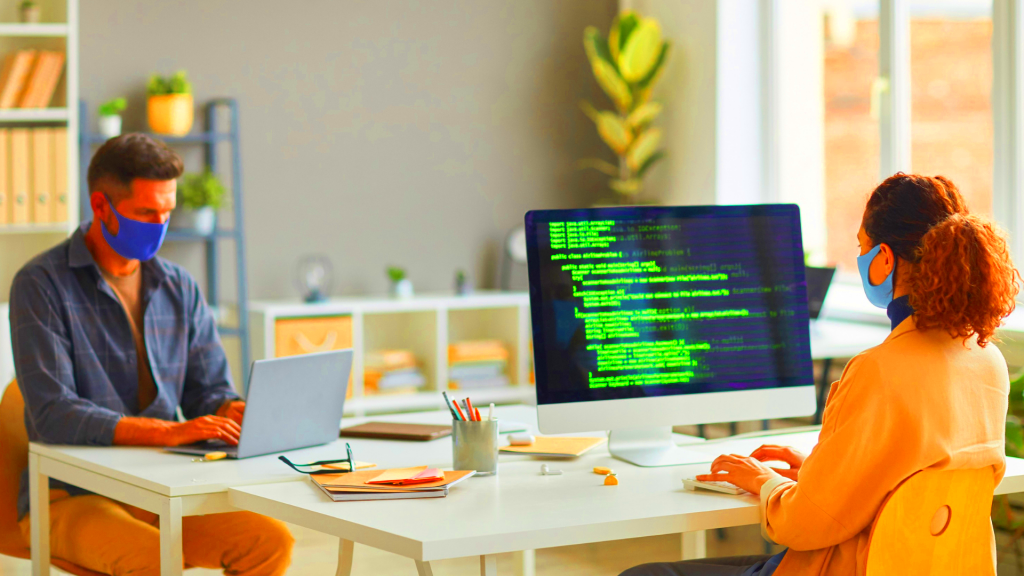
JavaScript offers several advantages when it comes to adding images dynamically to a website. Here are a few reasons why you should consider using JavaScript for this task:
- Improved User Experience: By using JavaScript, you can update or change images in response to user actions, such as clicking a button, hovering over an element, or even scrolling. This makes your website feel more interactive and responsive.
- Faster Loading Times: You can load images only when they are needed, reducing the initial load time of the page. This is especially helpful for image-heavy websites or those with multiple visuals.
- Better Control: JavaScript gives you full control over how and when images are added to the page, allowing for more advanced features like lazy loading or on-demand image changes.
- Optimized Content: You can load different images depending on various conditions, like screen size or user input. This ensures your site displays the most relevant image in any situation.
These reasons make JavaScript an excellent tool for creating dynamic and engaging website content that improves the overall user experience.
Steps to Add an Image Using JavaScript
Now that we understand the benefits of using JavaScript for dynamic images, let’s walk through the steps to add an image using JavaScript. It's easier than you might think! Follow these steps to add images to your webpage:
- Step 1: Create the Image Element
First, we need to create a new image element using JavaScript. You can do this by using thedocument.createElement()
method. This method will allow you to create animg
tag dynamically in your code. - Step 2: Set the Image Source
Once the image element is created, the next step is to set the source of the image. This can be done by assigning the image URL to thesrc
attribute of the image element. - Step 3: Add Alt Text
It’s important to provide alt text for images to ensure accessibility and help with SEO. You can set thealt
attribute to describe the image content. - Step 4: Append the Image to the DOM
Finally, after creating the image element and setting its attributes, you need to add it to the webpage. You can do this by usingappendChild()
orinsertBefore()
methods to append the image to a specific part of the DOM.
Here’s an example code snippet that demonstrates these steps:
// Create the image element
let img = document.createElement('img');
// Set the source of the image
img.src = 'https://example.com/image.jpg';
// Set the alt text
img.alt = 'A beautiful landscape';
// Append the image to the body of the page
document.body.appendChild(img);
By following these simple steps, you can easily add images to your website dynamically using JavaScript, making your content more interactive and flexible.
Using JavaScript to Add an Image to HTML
Adding an image to HTML using JavaScript is a straightforward process that gives you more control over your website content. Instead of manually placing an img
tag in your HTML code, JavaScript allows you to create and insert images dynamically as needed. This is particularly useful when you want to load images only after certain actions or events on the page, improving load times and user interaction.
To add an image, we use JavaScript to create an img
element, set the image source, and insert it into the webpage. The method is clean and efficient, helping to reduce clutter in the HTML code while allowing greater flexibility. Below are the steps to do this:
- Step 1: Create an Image Element
In JavaScript, you can create a newimg
element usingdocument.createElement('img')
. - Step 2: Set the Source
Once the element is created, set the source by assigning the image URL to thesrc
attribute of the image element. - Step 3: Set Alt Text
It’s important to include a description of the image for accessibility purposes. You can do this by setting thealt
attribute. - Step 4: Append to the DOM
Finally, append the image element to the webpage by usingdocument.body.appendChild(img)
, or insert it in a specific container on the page.
This approach gives you the flexibility to add images based on conditions or events, making your website more dynamic.
Adding Images Dynamically Based on User Interaction
One of the greatest advantages of using JavaScript to add images is that you can make the image loading process dependent on user interaction. Whether it's clicking a button, hovering over an element, or interacting with a form, you can trigger the addition of images dynamically. This enhances the user experience by providing more responsive and engaging content.
Here’s how you can set up image loading based on user actions:
- Click Events: Use an event listener to trigger an image addition when a user clicks a button or a link. This method is great for interactive galleries or slideshows.
- Hover Events: You can display different images when a user hovers over specific elements, such as buttons or images themselves, providing a more interactive design.
- Scroll Events: Adding images dynamically as the user scrolls down the page is known as lazy loading. This improves performance by loading images only when they are needed.
For example, consider a button that, when clicked, adds a new image to the page:
// Select the button
let button = document.getElementById('load-image');
// Add event listener to the button
button.addEventListener('click', function() {
// Create an image element
let img = document.createElement('img');
// Set the image source
img.src = 'https://example.com/new-image.jpg';
// Append the image to the page
document.body.appendChild(img);
});
This approach allows you to add content interactively, responding directly to the actions of your website visitors.
Working with External Image Links in JavaScript
When you add images using JavaScript, you don’t always have to use local files. External image links, such as those from a CDN (Content Delivery Network) or third-party sources, can be used to load images dynamically. This method allows you to use high-quality, externally hosted images without taking up space on your server or worrying about file management.
To work with external image links in JavaScript, you just need to provide the full URL of the image you want to display. This is no different from adding a local image, but you simply use the URL instead of a relative file path.
Advantages of using external image links include:
- Faster Load Times: Images hosted on CDNs are optimized for faster delivery, so they load more quickly compared to locally hosted images.
- Reduced Server Load: Hosting images externally offloads the bandwidth and storage needs from your server, improving overall performance.
- Easy Image Updates: By linking to external sources, you can easily update images without touching your code. Changes made on the external server will automatically reflect on your website.
Here’s an example of adding an image from an external source:
// Create an image element
let img = document.createElement('img');
// Set the source to an external image URL
img.src = 'https://examplecdn.com/image.jpg';
// Append the image to the page
document.body.appendChild(img);
By using external image links, you can efficiently manage images on your website, reduce server load, and keep your content fresh.
Optimizing Image Loading for Faster Performance
Optimizing image loading is crucial for improving website performance, particularly when you're using JavaScript to load images dynamically. Slow image loading can lead to a poor user experience, increased bounce rates, and lower search engine rankings. Thankfully, JavaScript offers several techniques to help you optimize image loading, ensuring that your website runs smoothly and efficiently.
Here are some tips to optimize image loading using JavaScript:
- Lazy Loading: Lazy loading is a technique that delays loading images until they are about to appear in the viewport. This reduces the initial load time and saves bandwidth, especially on pages with lots of images. You can easily implement lazy loading by setting the
loading="lazy"
attribute on the image elements or using JavaScript to detect when an image enters the viewport. - Responsive Images: To ensure that images load quickly on all devices, use the
srcset
attribute to provide multiple image sizes based on the screen resolution and device type. This ensures that users download the most appropriate image size for their screen, reducing unnecessary data usage. - Image Compression: Compressing images before adding them to your website can significantly reduce their file size without sacrificing quality. Tools like TinyPNG or ImageOptim can help you compress images before uploading them to your server or CDN.
- Use of WebP Format: WebP is an image format that provides better compression and quality compared to JPEG and PNG. Consider using WebP images for improved performance, especially for users with slower internet connections.
- Preload Important Images: If there are images critical to the user experience, such as a hero image or the first image in a slideshow, you can preload them using JavaScript. This ensures they are loaded as soon as possible, improving perceived performance.
By implementing these optimization techniques, you can ensure that images load quickly and smoothly, leading to a better user experience and improved website performance.
Common Mistakes to Avoid When Adding Images with JavaScript
While adding images dynamically with JavaScript can enhance your website’s interactivity and user experience, it’s important to avoid common mistakes that could negatively impact performance or usability. Here are some mistakes to watch out for:
- Forgetting Alt Text: Every image should include an
alt
attribute for accessibility purposes and SEO benefits. Omitting alt text can make your website less accessible to users with disabilities, and can hurt your search engine ranking. - Not Handling Image Errors: It’s important to handle errors when loading images. If an image fails to load, it can break the layout of your page. Use the
onerror
event in JavaScript to provide a fallback image or message, ensuring that your website remains functional even when image links are broken. - Overloading the Page with Too Many Images: Adding too many images dynamically can slow down your page, especially if they are large files or not optimized. Always be mindful of how many images you load at once and use techniques like lazy loading to delay the loading of offscreen images.
- Not Preloading Critical Images: If certain images are essential for the initial user experience, such as the logo or hero images, they should be preloaded to ensure they appear quickly when the page loads. Failing to do this can cause delays in the visual experience for users.
- Not Using Appropriate Image Formats: Using the wrong image format can result in unnecessarily large file sizes. For example, using PNG for images that don’t require transparency can be inefficient. Always choose the most suitable format for your images (e.g., JPEG for photos, PNG for transparent images, WebP for performance).
Avoiding these mistakes will help you create a smoother and more efficient user experience when dynamically adding images to your website.
FAQ
What is lazy loading and why is it important?
Lazy loading is a technique that delays the loading of images until they are needed, meaning they only load when they are about to enter the user's viewport. This helps improve page load times, reduce bandwidth usage, and enhance the overall performance of the website.
How can I make my images responsive using JavaScript?
You can make images responsive by using the srcset
attribute, which allows you to specify different image sizes for different screen resolutions and device widths. JavaScript can also dynamically set the src
attribute based on the user’s device to ensure the right image size is loaded.
How can I handle image loading errors in JavaScript?
You can handle image loading errors by using the onerror
event in JavaScript. This allows you to specify a fallback image or display an error message when an image fails to load.
What is the best image format to use for faster loading?
The best image format depends on the type of image. For photographs, JPEG is ideal due to its good compression. For images that need transparency, PNG is a good choice. For faster load times and better quality, consider using WebP, which is supported by most modern browsers.
Conclusion
Incorporating JavaScript to dynamically add images to your website can significantly enhance user experience and performance. By following best practices like lazy loading, image compression, and using responsive images, you can ensure that your website is not only visually appealing but also optimized for speed. Avoiding common mistakes like neglecting alt text or failing to handle image errors can prevent potential issues that affect both accessibility and functionality.
By leveraging the power of JavaScript, you can create a more engaging, responsive, and efficient website that meets the needs of your users, while also improving page load times and reducing unnecessary resource consumption. Whether you're adding images based on user interactions, using external image links, or optimizing your images for faster performance, JavaScript offers a flexible solution for managing dynamic content.
Ultimately, with the right strategies and careful attention to detail, you can make sure that your website remains fast, user-friendly, and visually engaging for everyone who visits.