Inverting image colors is a simple yet powerful technique that changes the color scheme of an image by replacing each color with its complementary counterpart. It’s like flipping the colors to their opposites, turning bright colors into darker shades and vice versa. This technique is often used in graphic design, photo editing, and even in programming challenges during interviews. The ability to invert colors manually or through code can help solve various problems, especially when dealing with
In coding interviews, image color inversion can be a great way to test your problem-solving skills and your understanding of basic image manipulation algorithms. Whether you’re tasked with solving this problem in a technical interview or just exploring ways to edit images, understanding how to invert colors is an essential skill for developers and designers alike.
Why Inverting Image Colors Can Be Challenging
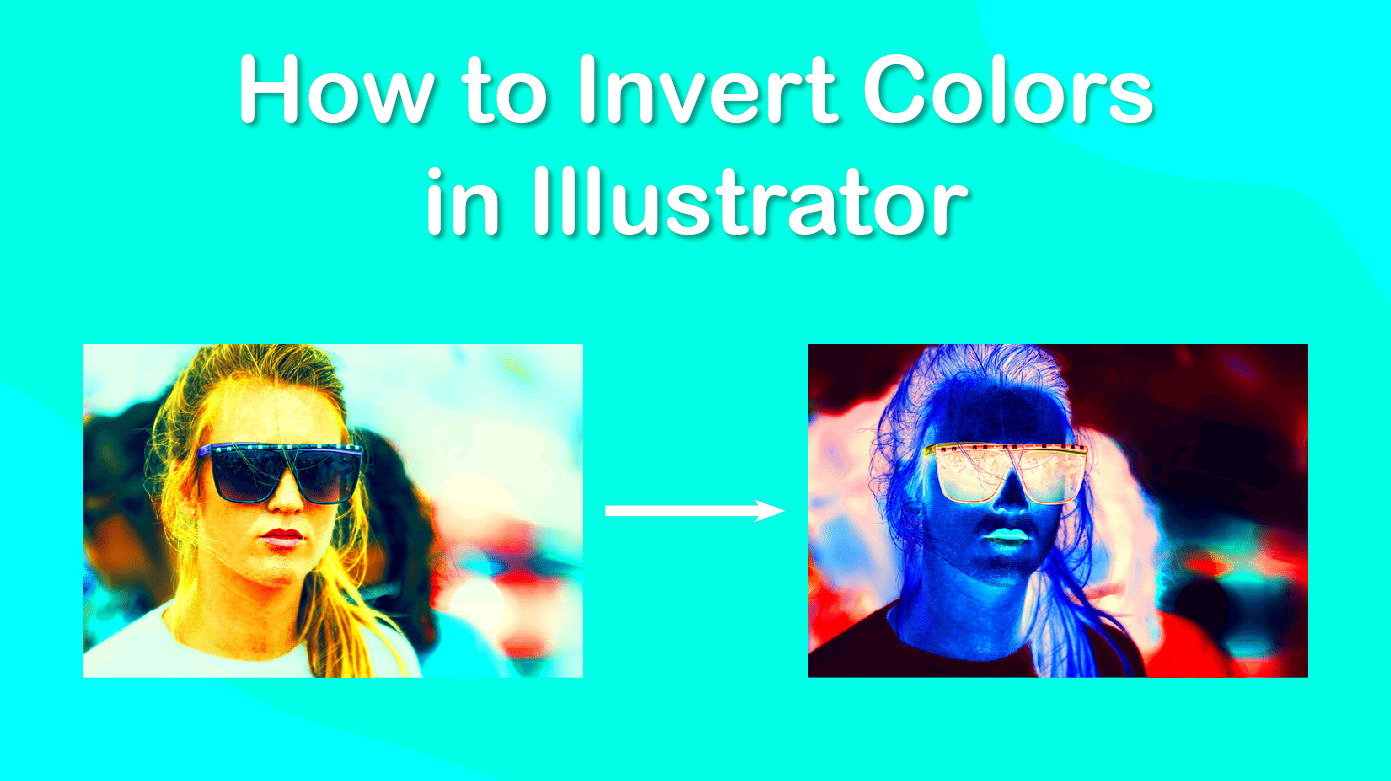
While inverting image colors seems like a simple task, it can pose some challenges, especially when done programmatically or when specific requirements need to be met. Here are a few reasons why this task can be tricky:
- Understanding Color Models: Images can be represented in different color models, such as RGB, CMYK, or HSL. Inverting colors requires knowing how these models work and how to properly transform colors in each model.
- Handling Transparency: Images with transparent backgrounds or alpha channels require special treatment to prevent inversion from affecting the transparency.
- Performance Considerations: Inverting colors for large images or in real-time can be resource-intensive, so performance optimization is often a factor to consider.
- Managing Negative Color Results: In some cases, inverting colors may result in unexpected visual outcomes, especially with certain color palettes or image content.
Despite these challenges, inverting colors remains an important task for developers and designers, and knowing how to approach it with the right techniques can help overcome these obstacles efficiently.
Also Read This: Solutions to Common Problems When Unable to Change the Thumbnail on Your YouTube Short
Steps to Invert Image Colors Manually
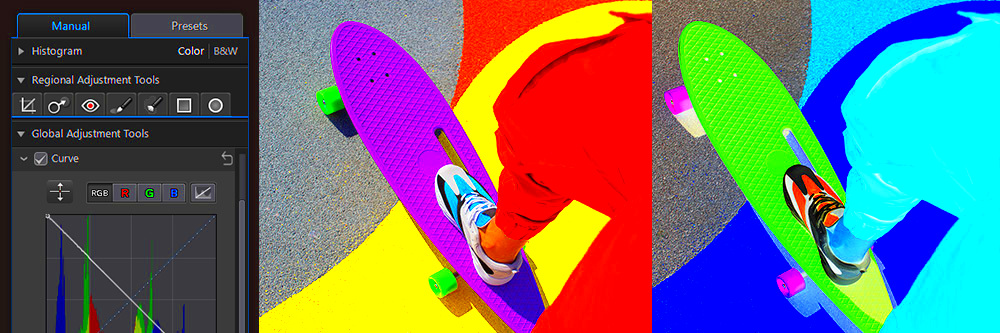
Inverting image colors manually involves a few straightforward steps. Whether you’re working with an image editing software or solving a coding challenge, here’s a general guide to get the job done:
- Identify the Color Model: First, identify the color model in which the image is represented. The most common one is the RGB (Red, Green, Blue) model. Each pixel’s color is defined by its red, green, and blue components.
- Find the Complementary Colors: To invert the colors, you need to find the complementary color for each component. For RGB, the complementary color is found by subtracting each color value from 255. For example, if the pixel is (100, 150, 200), its inverted color would be (155, 105, 55).
- Apply the Inversion: Once you have the complementary color for each pixel, apply the inversion by adjusting each pixel’s RGB values accordingly.
- Repeat for All Pixels: Continue the process for every pixel in the image until all colors are inverted. This can be done manually by editing each pixel or using an image editing tool.
If you're using programming to perform this task, you can write a simple function to automate this process. For example, in Python, you could use a loop to iterate over the pixel values of the image, invert them, and generate the new image. Here's a basic outline of how you could approach it:
# Pseudocode example for inverting an image for each pixel in image: red, green, blue = pixel inverted_pixel = (255-red, 255-green, 255-blue) set new pixel value in new image
By following these steps, you can easily invert the colors of any image manually or with code, which can be useful for various image-processing tasks, from design to programming challenges in interviews.
Also Read This: Here’s How to Download Video Memes from iFunny to Your Phone
How to Use Programming to Invert Image Colors
Inverting image colors using programming is a great way to automate the process, especially when dealing with large sets of images or when you need to integrate it into an application. By using programming languages like Python, you can quickly manipulate images by applying color inversion algorithms. Here’s a basic overview of how you can approach this task:
To get started, you’ll need an image processing library such as Python’s Pillow or OpenCV. These libraries allow you to open, manipulate, and save images with just a few lines of code. Let’s walk through the general process:
- Install a Library: Begin by installing a library like Pillow. You can install it using pip:
pip install pillow
- Open the Image: Load the image you want to invert into memory.
from PIL import Image
image = Image.open("path_to_image.jpg")
- Invert the Colors: Use the image’s RGB values to invert the colors. With Pillow, this can be done with the
ImageOps.invert()
function.from PIL import ImageOps
inverted_image = ImageOps.invert(image.convert("RGB"))
- Save the Image: After inverting the colors, save the new image to your computer.
inverted_image.save("inverted_image.jpg")
This method works well for basic image manipulation. However, if you need to handle more complex tasks such as real-time processing or working with videos, you might want to explore more advanced libraries like OpenCV or NumPy. These libraries offer faster and more efficient ways to manipulate images at scale.
Also Read This: How to Convert Dailymotion Videos to MP4 Easily
Tools for Inverting Image Colors
If you don’t want to write code to invert image colors, there are plenty of online tools and software that can help. These tools are user-friendly and allow you to invert colors in just a few clicks. Here are some popular tools you can use:
- Photopea: A free, online image editor that closely mimics Photoshop’s functionality. Simply upload your image, and use the “Invert” filter under the “Image” menu to reverse the colors.
- Adobe Photoshop: The industry-standard image editing software offers a range of color manipulation tools, including the ability to invert colors. You can use the “Invert” option in the Adjustments panel to achieve the effect.
- GIMP: A free and open-source alternative to Photoshop. GIMP offers an easy way to invert colors using the “Colors” menu, followed by selecting “Invert” to quickly reverse the image’s colors.
- Online Image Color Inverters: Websites like imgonline.com.ua and photopea.com let you upload an image and apply color inversion with no software installation required.
These tools are great for casual users who need a quick solution. They are perfect when you want to invert a single image without getting into the complexities of coding or dealing with large batches of images. Some tools even offer additional features, like adjusting the intensity of the color inversion or applying it to specific parts of the image.
Also Read This: Discover Stunning and Unique Vectors on VectorStock
Common Mistakes to Avoid
Inverting image colors is a straightforward process, but there are several mistakes that people often make, especially when they are new to image manipulation or coding. Let’s go over some of these common mistakes and how to avoid them:
- Not Converting to RGB First: In many cases, images are not initially in RGB format (for example, some images are in CMYK or grayscale). Inverting colors in an unsupported color space can lead to unexpected results. Always convert images to RGB before performing color inversion.
- Forgetting Transparency: When dealing with images that have an alpha (transparency) channel, such as PNG files, make sure the transparency is preserved. Inverting the color values without considering the alpha channel can lead to strange results where the transparency is lost or altered.
- Not Considering Performance: Inverting colors for large images can be resource-intensive. When working with large batches of images or large image files, consider optimizing your code to process images efficiently or resize the image before performing the inversion.
- Overusing Inversion: Inverting colors works well for certain tasks, but it’s not always the best solution. In some cases, the inversion may make the image harder to understand or aesthetically unpleasing. Use it only when appropriate, and make sure it adds value to the task or design.
- Ignoring the Visual Impact: Inverting colors may result in a visually jarring or uncomfortable image, especially when used with high contrast or neon colors. Consider adjusting the brightness, saturation, or contrast after the inversion to make the result more visually appealing.
By being mindful of these common mistakes, you can avoid frustration and ensure that your color inversion efforts result in clean and effective images.
Also Read This: Easy Guide to Download Videos from Dailymotion
Benefits of Mastering Image Color Inversion for Interviews
Mastering the technique of inverting image colors can offer significant advantages, particularly when preparing for technical interviews. It’s a skill that not only demonstrates your understanding of image processing but also highlights your ability to solve problems efficiently. Here’s why this skill is valuable:
- Showcases Problem-Solving Ability: In interviews, the ability to manipulate images and solve problems related to them can set you apart. Mastering color inversion shows that you can break down a complex problem into manageable steps and implement a solution.
- Demonstrates Knowledge of Algorithms: The process of inverting colors is an algorithmic challenge, and understanding how to do it using different color models (like RGB or CMYK) shows that you grasp important concepts in algorithms and image manipulation.
- Improves Coding Efficiency: Knowing how to invert image colors with code not only prepares you for technical interviews but also helps with day-to-day coding tasks, improving your speed and efficiency when solving related problems.
- Highlights Attention to Detail: Inverting image colors might seem simple, but getting the details right—especially when handling edge cases like transparency or performance—demonstrates a keen eye for detail and a thorough understanding of coding practices.
- Broadens Your Skill Set: Having this skill under your belt opens doors to a variety of image processing and computer vision tasks. It’s a versatile skill that can be useful in many industries, from web development to machine learning.
By mastering color inversion, you not only prepare yourself for technical challenges in interviews but also develop a deeper understanding of how images can be manipulated in code, which is a valuable asset in today’s tech-driven world.
Also Read This: Learn How to Automatically Trace an Image in Procreate
FAQ
Here are some common questions people ask about inverting image colors and how it can be used in coding and interviews:
- Q: What is the best way to invert an image's colors?
A: The easiest way to invert an image’s colors is by using image processing libraries like Pillow (Python) or built-in tools like Photoshop. For coding, you can apply color inversion by subtracting the RGB values from 255. - Q: Does inverting an image affect its quality?
A: Inverting colors doesn’t affect the image's resolution or pixel quality, but it can alter the image’s appearance significantly, which may not always be desirable. It’s important to test the results and adjust as needed. - Q: Can I invert image colors in real-time applications?
A: Yes, you can. For real-time applications such as web or mobile apps, you can use JavaScript or Python to apply color inversion on the fly, ensuring smooth user interactions. - Q: Should I use color inversion in every project?
A: While color inversion is useful for certain tasks, it’s not suitable for every project. Use it when it enhances the overall design or functionality of your application or design. - Q: Is it possible to invert specific parts of an image?
A: Yes, you can invert specific regions of an image by applying the inversion algorithm only to those sections. This is often done in more advanced image editing and processing tasks.
Conclusion
Inverting image colors is more than just a simple technique—it’s a powerful tool that can be used in a variety of situations, from graphic design to technical interviews. Mastering this skill not only gives you an edge in problem-solving but also broadens your understanding of image processing and algorithms. Whether you are preparing for a coding interview or working on a design project, the ability to manipulate image colors efficiently can enhance your workflow and demonstrate your technical capabilities.
By learning how to invert image colors manually or programmatically, you add a versatile skill to your toolkit. Whether you are working with Python, Photoshop, or online tools, knowing when and how to use color inversion will make you a more well-rounded professional and prepare you for a variety of challenges in both interviews and real-world applications.