Converting HTML to images in Java can be a highly useful tool for developers working with web content. It allows the transformation of web pages or HTML code into image files, making it easier to display the content in various formats, store it for later use, or share it as static content. Java provides powerful libraries and tools to make this process efficient and effective. Whether you are working on web scraping, generating previews, or automating screenshots, knowing how to convert HTML to images can save you time and effort.
Understanding the Process of HTML to Image Conversion
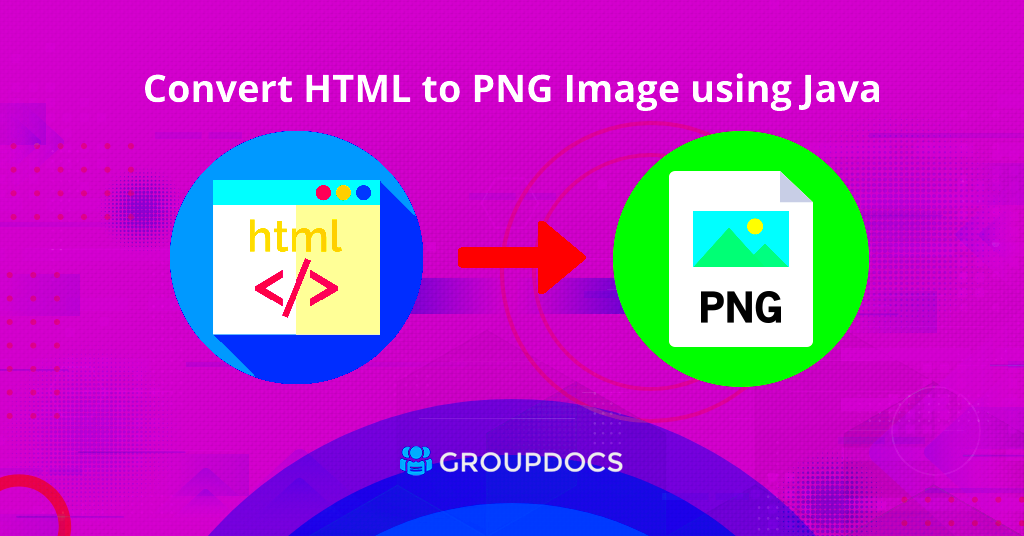
When converting HTML to images, the goal is to render a web page (or its elements) as an image. This process typically involves using a web browser engine or a rendering tool to interpret the HTML content and then output it as an image file (such as PNG, JPG, or BMP). Here’s a breakdown of how this works:
- HTML Rendering: The HTML code is parsed, and the elements are rendered as a visual representation.
- Layout Handling: CSS (Cascading Style Sheets) and JavaScript are processed to ensure the page appears as intended.
- Image Output: Once the page is rendered, a screenshot or image is captured, representing the entire page or a portion of it.
This process is useful for generating static snapshots of dynamic content, like web applications or interactive designs. It’s also great for taking snapshots of web pages that need to be stored, shared, or analyzed without retaining the live version of the page.
Tools and Libraries Available for HTML to Image Conversion in Java
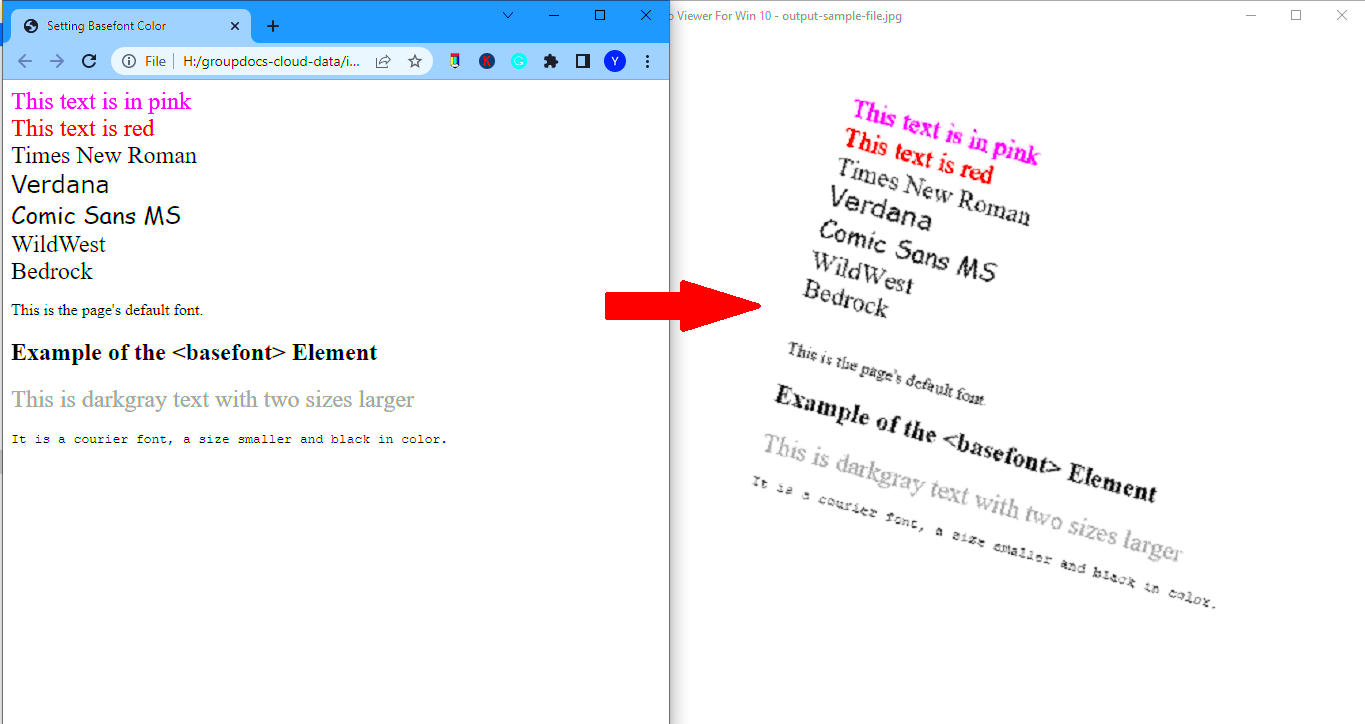
Java provides several tools and libraries that can assist in converting HTML to images. These tools simplify the conversion process by handling the technical complexities of rendering and image creation. Below are some of the most commonly used libraries for this task:
- JavaFX: JavaFX is a powerful framework for building GUI applications, and it includes features for rendering HTML to images. With JavaFX, you can load HTML content into a web view and capture it as an image.
- JxBrowser: This is a commercial library that allows you to embed a Chromium-based browser inside a Java application. It can render HTML pages and capture screenshots, making it ideal for HTML to image conversion.
- HtmlUnit: HtmlUnit is a headless browser used for web scraping, testing, and rendering HTML content. While it doesn’t provide direct image capture, it can be used alongside Java libraries to render HTML and generate images.
- Selenium: Though Selenium is primarily used for browser automation, it can also be used to render a web page and take screenshots of the page in various formats.
- WkHtmlToImage: This command-line tool, when integrated with Java, allows you to convert HTML files into images with high accuracy and quality.
These libraries and tools offer a range of features depending on your needs, whether it’s a simple conversion or a more complex rendering with interactivity and dynamic content.
Setting Up Your Java Environment for HTML to Image Conversion
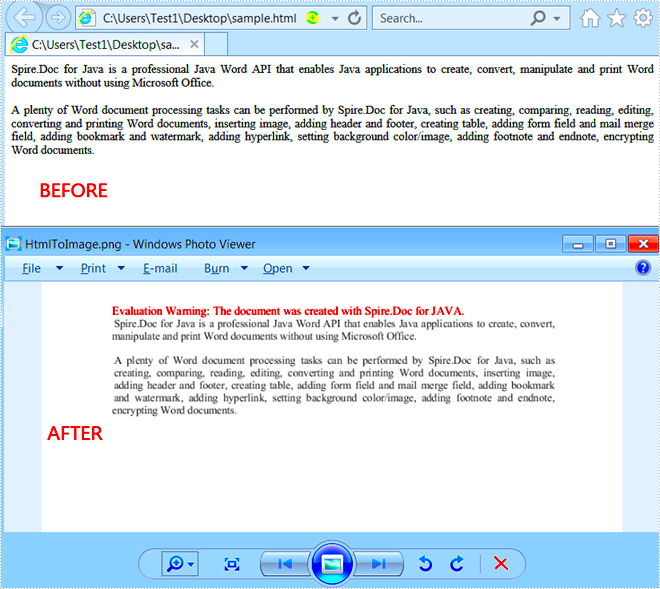
Before you can start converting HTML to images in Java, you need to set up your development environment. This process involves installing the necessary tools, libraries, and dependencies to ensure your Java project can successfully render HTML content as an image. Here's how you can get started:
- Install Java Development Kit (JDK): Make sure you have the latest version of the JDK installed on your system. This is essential for compiling and running Java programs. You can download it from the official Oracle website or other trusted sources.
- Set Up an Integrated Development Environment (IDE): Choose an IDE that suits your needs. Popular choices for Java development include IntelliJ IDEA, Eclipse, and NetBeans. These IDEs make coding and debugging easier.
- Include Required Libraries: Depending on the tool or library you plan to use for HTML to image conversion (like JavaFX, JxBrowser, or Selenium), you'll need to include these libraries in your project. For example, you can add them to your project as dependencies in Maven or Gradle.
- Configure WebDriver for Selenium (if using): If you're using Selenium, make sure you configure the appropriate WebDriver (like ChromeDriver or GeckoDriver) for browser automation.
- Test the Setup: Once everything is installed, run a simple Java program to verify that the setup works correctly. You can try loading a basic HTML page and see if the rendering engine is working as expected.
After completing these steps, you’ll be ready to start converting HTML into images using your Java environment. Ensuring everything is set up correctly will save you a lot of troubleshooting time later on.
Code Implementation for HTML to Image Conversion in Java
Now that your environment is set up, it's time to implement the code that will convert HTML to images. Below is an overview of how you can approach the implementation, using libraries such as JavaFX or Selenium for rendering and taking screenshots:
- Using JavaFX: JavaFX includes a WebView component, which you can use to load and render HTML content. Here’s a basic outline of the steps:
- Create a JavaFX application and initialize a WebView.
- Load the desired HTML content into the WebView.
- Capture the content as an image using the
Snapshot
method of the WebView.
- Using Selenium WebDriver: If you're automating a browser with Selenium, you can take screenshots of the rendered HTML page. Here's how you can do it:
- Initialize a WebDriver instance (e.g., ChromeDriver).
- Open the desired URL or load the HTML content into the browser.
- Use the
getScreenshotAs
method to capture the entire page as an image.
- Code Example: Here’s a basic code example using JavaFX:
import javafx.application.Application; import javafx.scene.Scene; import javafx.scene.image.WritableImage; import javafx.scene.web.WebView; import javafx.stage.Stage; public class HtmlToImage extends Application { @Override public void start(Stage primaryStage) { WebView webView = new WebView(); webView.getEngine().load("https://www.example.com"); WritableImage image = webView.snapshot(null, null); // Save or process the image as needed } public static void main(String[] args) { launch(args); } }
This is just a basic implementation. You can extend this by adding error handling, dynamic page loading, or more complex HTML content rendering.
Handling Different Image Formats in HTML to Image Conversion
When converting HTML to images, you may want to save the output in different image formats, depending on your needs. Java allows you to handle multiple formats like PNG, JPG, and BMP, among others. Below are the main things to consider:
- PNG Format: PNG is a lossless format and is commonly used for images that need high quality and transparency. It’s ideal for images that include logos, charts, and graphics with text.
- JPG/JPEG Format: JPG is a lossy compression format that reduces file sizes at the cost of some image quality. It’s suitable for photographs or images with lots of colors and gradients. This format can help reduce the storage requirements of large images.
- BMP Format: BMP files are uncompressed and usually large in size. While not as commonly used as PNG or JPG, it may be necessary for certain applications or compatibility with older systems.
- GIF Format: GIF is commonly used for simple images and animations. However, it is limited to 256 colors, so it’s not ideal for complex images.
To handle different formats in Java, you can use the ImageIO
class, which supports reading and writing various image types:
- Saving in PNG:
ImageIO.write(image, "PNG", new File("output.png"));
- Saving in JPG:
ImageIO.write(image, "JPG", new File("output.jpg"));
- Handling Compression: If you need to adjust the quality of JPG images, you can use ImageWriter to set compression levels.
By understanding how to handle different formats, you can ensure that the HTML to image conversion meets your requirements, whether it’s for web use, printing, or storage.
Best Practices for HTML to Image Conversion in Java
When converting HTML to images in Java, following best practices can ensure better performance, higher-quality output, and smoother results. Here are some key tips to consider when implementing this process:
- Optimize the HTML Content: Simplify your HTML code as much as possible before converting it to an image. This helps improve rendering times and reduces errors during the conversion. Avoid unnecessary scripts, large images, or complex animations that may slow down the process.
- Use a Reliable Library: Choose a robust and well-documented library for rendering HTML content. Libraries like JavaFX or Selenium can give you better control over the conversion process and ensure that the output matches your expectations.
- Control Image Quality: Depending on the format you’re saving the image in (e.g., PNG, JPG), adjust the quality settings accordingly. If using JPG, for instance, reduce the quality slightly to improve file size without significant quality loss.
- Handle Dynamic Content Carefully: If your HTML includes dynamic content like JavaScript, make sure to fully load the page before capturing the image. This ensures that all elements (like images, buttons, etc.) are rendered and included in the final output.
- Set Proper Resolution: Ensure the resolution of the image is appropriate for your needs. For high-quality prints or detailed displays, increase the resolution. For smaller previews, lower the resolution to save storage space and loading time.
- Test Across Different Browsers: Different browsers render HTML in slightly different ways. If using a browser-based tool like Selenium or JxBrowser, test across multiple browsers to ensure consistent output.
Following these best practices will help you achieve optimal results, whether you are generating previews, screenshots, or static web content images in Java.
Common Issues and How to Solve Them
While converting HTML to images in Java, developers may encounter various challenges. Below are some common issues and their solutions to help ensure a smooth conversion process:
- Rendering Issues: Sometimes, the HTML content may not render as expected, leading to missing or distorted elements in the image.
- Solution: Make sure all external resources like CSS, JavaScript, and images are correctly loaded before capturing the image. Use the
WebView.setOnReady
orWebDriver.wait()
method to ensure the page is fully loaded.
- Solution: Make sure all external resources like CSS, JavaScript, and images are correctly loaded before capturing the image. Use the
- Slow Rendering: Converting complex HTML pages with heavy styles or content can take a lot of time, causing delays or crashes.
- Solution: Optimize the HTML content by removing unnecessary elements or simplifying CSS. You can also try reducing the resolution for faster processing.
- Low Image Quality: The image output may sometimes be blurry or pixelated, especially when scaling the HTML content to a different size.
- Solution: Increase the resolution of the WebView or use the
WritableImage
class in JavaFX to capture a higher-quality image. Avoid resizing images in the post-processing stage if possible.
- Solution: Increase the resolution of the WebView or use the
- Image Format Issues: Certain image formats (e.g., PNG, JPG) may not be supported by all tools, or they may not provide the best output for your needs.
- Solution: Test different formats and choose the one that best suits your requirements. PNG is ideal for preserving quality, while JPG is better for reducing file size.
- JavaScript and Dynamic Content: If the HTML includes JavaScript-driven content (e.g., dropdowns, animations), this may not render correctly when captured as an image.
- Solution: Ensure that all JavaScript functions have been executed before taking the screenshot. Use tools like
WebDriverWait
in Selenium or set a delay in JavaFX to allow scripts to load.
- Solution: Ensure that all JavaScript functions have been executed before taking the screenshot. Use tools like
By addressing these common issues and understanding their solutions, you can make the HTML to image conversion process more efficient and effective in Java.
FAQ
Here are some frequently asked questions related to converting HTML to images in Java:
- What Java libraries can be used to convert HTML to images?
Popular libraries for this purpose include JavaFX, Selenium, JxBrowser, and HtmlUnit. Each offers different features, so choose one based on your specific needs.
- Can I capture dynamic content (like JavaScript) in HTML when converting it to an image?
Yes, you can. However, you must ensure that all dynamic content is fully loaded before capturing the screenshot. Use appropriate waits in libraries like Selenium or JavaFX.
- How can I improve the image quality during conversion?
To improve image quality, make sure you use a high resolution for the image capture. If using JPG format, adjust the quality settings to balance image quality and file size.
- Which image format should I use for HTML to image conversion?
The best image format depends on your use case. PNG is ideal for images requiring transparency and higher quality, while JPG is better for reducing file sizes. BMP and GIF may be useful in specific scenarios, though less common.
- Can I convert an entire webpage, including images, to an image?
Yes, with the right libraries like Selenium or JavaFX, you can capture an entire webpage, including images and other assets, into a single image file.
Conclusion
Converting HTML to images in Java is a valuable technique for developers working with web content, offering a variety of practical uses like creating snapshots, generating static previews, or automating web scraping tasks. By understanding the process, choosing the right tools, and following best practices, you can achieve high-quality, efficient conversions. Whether you're using JavaFX, Selenium, or other libraries, this process allows you to capture dynamic and static HTML content as image files in various formats, like PNG, JPG, and more. With careful setup, attention to detail, and troubleshooting common issues, HTML to image conversion in Java becomes a powerful tool in any developer's toolkit. Always ensure to test across different browsers and environments for consistent results. In the end, HTML to image conversion is a skill worth mastering for anyone looking to work with web content outside of its usual HTML format.