Displaying images in Angular is an essential task in many web applications, whether you're building a gallery, a product showcase, or integrating with an API to display dynamic content. Angular, a powerful JavaScript framework, makes it relatively simple to handle data, including images, through services and components. By fetching image data from an API, you can display images dynamically in your Angular app without manually adding each image source.
In this guide, we’ll walk through how to display images from an API in your Angular application. We’ll cover everything from setting up your project to handling image display and performance optimization. This approach ensures your app remains responsive and scalable as the number of images grows.
Understanding the Basics of Angular and APIs
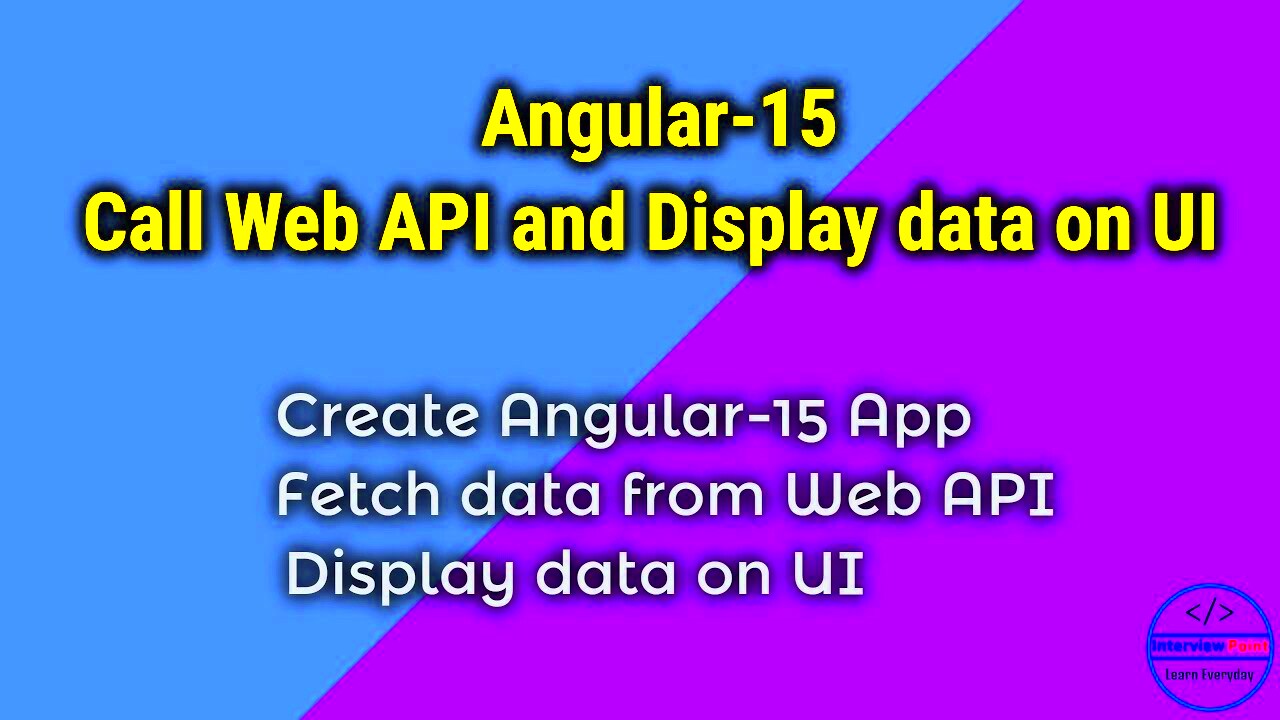
Before diving into the specifics of displaying images, let’s break down what you need to know about Angular and APIs.
Angular is a TypeScript-based open-source framework that simplifies the development of dynamic, single-page applications. It allows you to build applications that can retrieve data from various sources, including APIs, and display it on the web. The key to displaying images in Angular lies in understanding how data binding works between your components and services.
API stands for Application Programming Interface, and it’s a set of rules that allows different software components to communicate with each other. In this case, the API will send image data to your Angular application. The data can be in various formats like JSON, and it often includes URLs pointing to the location of the images on a server.
Here’s a quick rundown of the steps involved in displaying images in Angular:
- Make an API call to fetch image data
- Parse the data returned by the API
- Bind the image data to your Angular component for display
Understanding these basic concepts will give you a solid foundation for displaying images in your app, ensuring smooth integration between Angular and APIs.
Also Read This: Adobe Stock Images No Watermark: How to Use Them Legally
Setting Up Your Angular Project
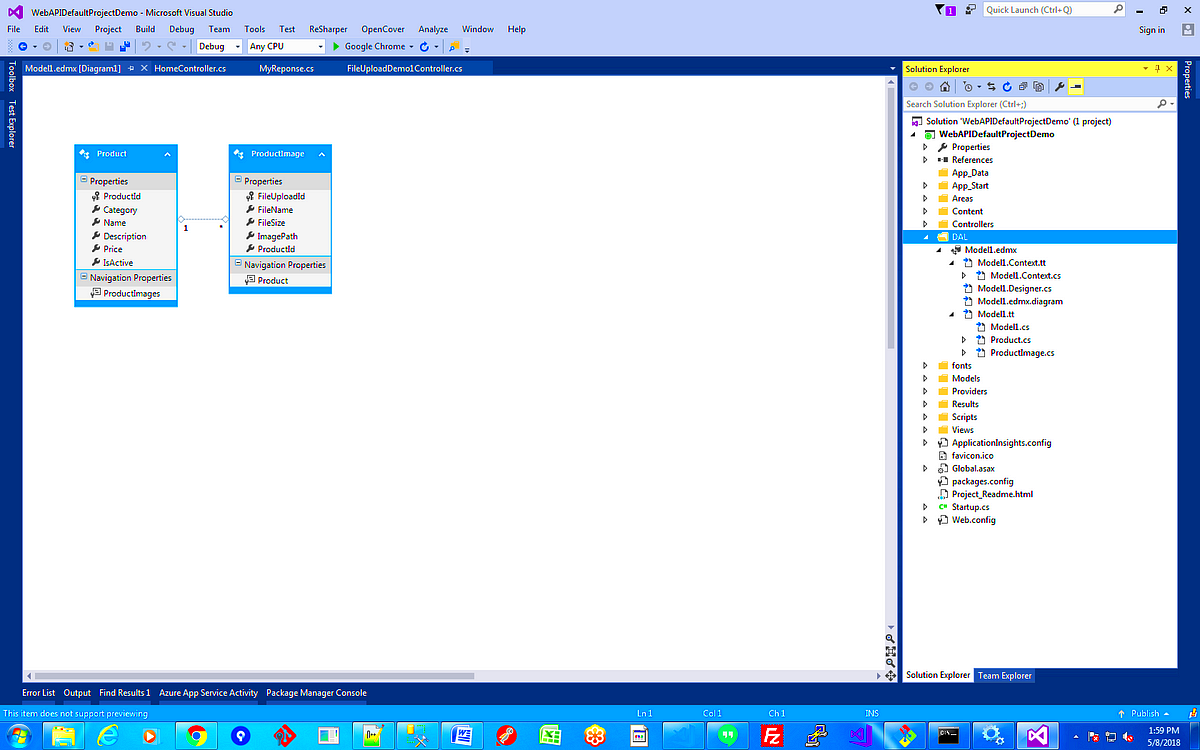
Before you start working with images in Angular, you need to set up a new Angular project. Let’s go through the steps needed to get your project ready for displaying images from an API.
Step 1: Install Angular CLI
If you haven’t already, you’ll need to install Angular CLI (Command Line Interface). This tool helps you create and manage Angular projects easily. You can install it globally on your machine using npm:
npm install -g @angular/cli
Step 2: Create a New Angular Project
Once Angular CLI is installed, create a new project by running the following command:
ng new image-display-app
This command will create a new directory with the necessary files and dependencies for your project. When prompted, choose options such as routing and stylesheets based on your preference.
Step 3: Navigate to Your Project Directory
Once the project is created, navigate into the project folder:
cd image-display-app
Step 4: Serve the Application
Now that your project is set up, serve the application locally using the Angular CLI. Run the following command:
ng serve
After a few moments, open your browser and navigate to http://localhost:4200 to see your new Angular app running.
At this point, your Angular environment is ready. The next steps will involve creating components, services, and integrating the API to display images. But first, let’s ensure you have everything set up correctly to handle the incoming data from the API.
Also Read This: Mastering Your Getty Images Login and Account Management
Creating a Service to Fetch Images from an API
Now that you have your Angular project set up, the next step is to create a service that will fetch image data from an API. Services in Angular are used to handle logic that is not directly related to the view, such as fetching data, processing it, or interacting with external APIs. By creating a service, we can keep our components clean and focused on displaying data, while the service handles the communication with the API.
Step 1: Generate a Service
First, you need to generate the service that will fetch the image data. Run this command in your terminal:
ng generate service image
This will create an image service file in your project. Open the image.service.ts file, where you'll write the logic for fetching image data from the API.
Step 2: Set Up HTTP Client Module
Angular uses the HTTP Client module to make API requests. You need to import and configure it in your app. In the app.module.ts file, add the following import:
import { HttpClientModule } from '@angular/common/http';
Then, add HttpClientModule to the imports array:
imports: [HttpClientModule]
Step 3: Write the Logic to Fetch Data
Now, in your image.service.ts file, you can use Angular’s HttpClient to make a GET request to the API that provides the image data. For example:
import { Injectable } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Observable } from 'rxjs'; @Injectable({ providedIn: 'root' }) export class ImageService { constructor(private http: HttpClient) { } fetchImages(): Observable { return this.http.get('https://api.example.com/images'); } }
In this code, we’re fetching images from an API endpoint. The response is returned as an observable, which you can subscribe to in your component to get the data.
With the service in place, your Angular app is now ready to fetch image data from an API!
Also Read This: Understanding iStock Subscription Costs and Plans for Every Need
Binding Image Data to Angular Components
Once you have your service set up to fetch image data from the API, the next step is to bind that data to an Angular component so that the images can be displayed in the view. In Angular, you can use data binding to dynamically display content in your HTML based on the data returned from the service.
Step 1: Import and Inject the Service
In the component where you want to display the images (let’s say app.component.ts), first import the ImageService and inject it into the constructor:
import { Component, OnInit } from '@angular/core'; import { ImageService } from './image.service'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements OnInit { images: any[] = []; constructor(private imageService: ImageService) {} ngOnInit() { this.imageService.fetchImages().subscribe(data => { this.images = data; }); } }
In this code, we are subscribing to the observable returned by the service’s fetchImages() method and storing the response (the image data) in the images array.
Step 2: Bind Data in the Template
Next, in the app.component.html file, you can loop through the images array and display the images using Angular’s *ngFor directive:
This will loop through all the images in the images array and display each one using its URL and title. Angular’s src attribute binding is used to set the image source dynamically.
Now, the images fetched from the API will be displayed in your Angular component, and the component will automatically update whenever the data changes.
Also Read This: Get to Know How Many Images Shutterstock Has
Handling Image Display with Angular Directives
Angular provides powerful directives like *ngFor and *ngIf that allow you to control the display of elements based on data. When working with images, these directives become very useful for displaying multiple images or handling edge cases such as broken links or missing images.
*ngFor Directive
The *ngFor directive is perfect for looping over an array of image data and displaying multiple images. In the previous section, we used *ngFor to loop through the images array and display each image. Here’s a quick refresher:
This loop will create an img tag for each item in the array, dynamically binding the image URL and alt text to the properties of the image object.
*ngIf Directive for Conditional Display
Sometimes, you may need to display images only under certain conditions. For example, you might want to show a loading spinner until the images are fully loaded, or display a placeholder if an image is missing. This is where *ngIf comes in handy. You can use it to conditionally render elements based on the availability of data:
![]()
No images available at the moment.
In this example, the images are only displayed if the images array has data. If the array is empty, the message "No images available" is shown instead.
Handling Broken Images
To improve user experience, you may want to display a default or fallback image when a particular image fails to load. You can do this using Angular’s (error) event on the img tag:
In the component’s method, you can handle the error and replace the source with a fallback image:
onError(event: any) { event.target.src = 'path/to/fallback-image.jpg'; }
By using these Angular directives, you can manage how images are displayed, handle conditional rendering, and ensure a smooth experience even when things don’t go as expected.
Also Read This: How to Purchase a Getty Image for Your Projects
Optimizing Image Loading for Performance
When displaying images in an Angular application, performance is a key consideration, especially if you are loading large or many images from an API. Optimizing image loading not only improves the user experience but also ensures that your application loads faster, even on slower networks. There are several strategies you can implement to optimize image loading in Angular.
1. Lazy Loading Images
Lazy loading is a technique where images are only loaded when they come into view on the user’s screen. This improves the initial load time of the application since only the images that are visible initially are loaded. You can use the Intersection Observer API or a third-party library to achieve lazy loading in Angular:
import { Directive, ElementRef, HostListener } from '@angular/core'; @Directive({ selector: '[appLazyLoad]' }) export class LazyLoadDirective { constructor(private el: ElementRef) {} @HostListener('window:scroll', []) onWindowScroll() { const image = this.el.nativeElement; if (this.isInViewport(image)) { image.src = image.dataset.src; } } private isInViewport(image: HTMLImageElement) { const rect = image.getBoundingClientRect(); return rect.top >= 0 && rect.bottomIn the template, use the directive like this:
![]()
2. Image Compression
Another way to optimize images is by compressing them. Tools like TinyPNG or ImageOptim can help reduce the file size without compromising the quality too much. This is particularly helpful for large images, as smaller files load faster and use less bandwidth.
3. Responsive Images
Using responsive images ensures that your app serves appropriately sized images depending on the user’s screen resolution and device. You can use the srcset attribute to specify different image sizes for different display scenarios:
![]()
By implementing these strategies, you can significantly improve the performance of your Angular application, making it faster and more efficient for your users.
Also Read This: Locating an Image ID: A Simple Guide
Conclusion: Bringing It All Together
Displaying images in Angular from an API is a straightforward process when you follow the right steps. From setting up the Angular project to creating a service for fetching image data and binding that data to components, Angular makes it easy to integrate images into your web application. Along the way, we also discussed the importance of optimizing image loading for performance, using strategies like lazy loading, image compression, and responsive images.
By following this guide, you now have a solid foundation to create image-rich Angular applications that are both dynamic and performant. With Angular’s powerful features and a little attention to detail, you can build applications that provide a great user experience even when handling large numbers of images or high-resolution media.
Remember, performance optimization is an ongoing process. Regularly assess your app's performance and keep an eye on new techniques and best practices for image handling to ensure your app remains fast and efficient.
FAQ
Q: How can I improve the loading speed of my images in Angular?
A: To improve image loading speed, you can implement lazy loading, which only loads images when they are in the viewport. You can also compress images to reduce their file size and use responsive images to serve the appropriate image size based on the device's screen resolution.
Q: Can I fetch images from multiple APIs in Angular?
A: Yes, you can make multiple HTTP requests to different APIs and bind the results to your Angular components. You can either call the APIs sequentially or use forkJoin or mergeMap to handle multiple asynchronous requests simultaneously.
Q: What is the best format for images in Angular?
A: The best format depends on the type of images you are working with. For photographs, JPEG is often preferred due to its good compression. For images with transparency or simpler graphics, PNG or WebP may be more suitable. WebP is also a great choice for modern browsers as it offers superior compression.
Q: How do I handle broken image links in Angular?
A: You can handle broken image links using the (error) event on the img tag in your template. This allows you to provide a fallback image or show a message when an image fails to load:
![]()
In your component method:
onError(event: any) { event.target.src = 'path/to/fallback-image.jpg'; }