Python is a fantastic language for handling images. Whether you're analyzing photos, creating graphics, or working with machine learning, displaying images is an essential skill. In this post, we will explore various methods to open and show
Setting Up Your Python Environment
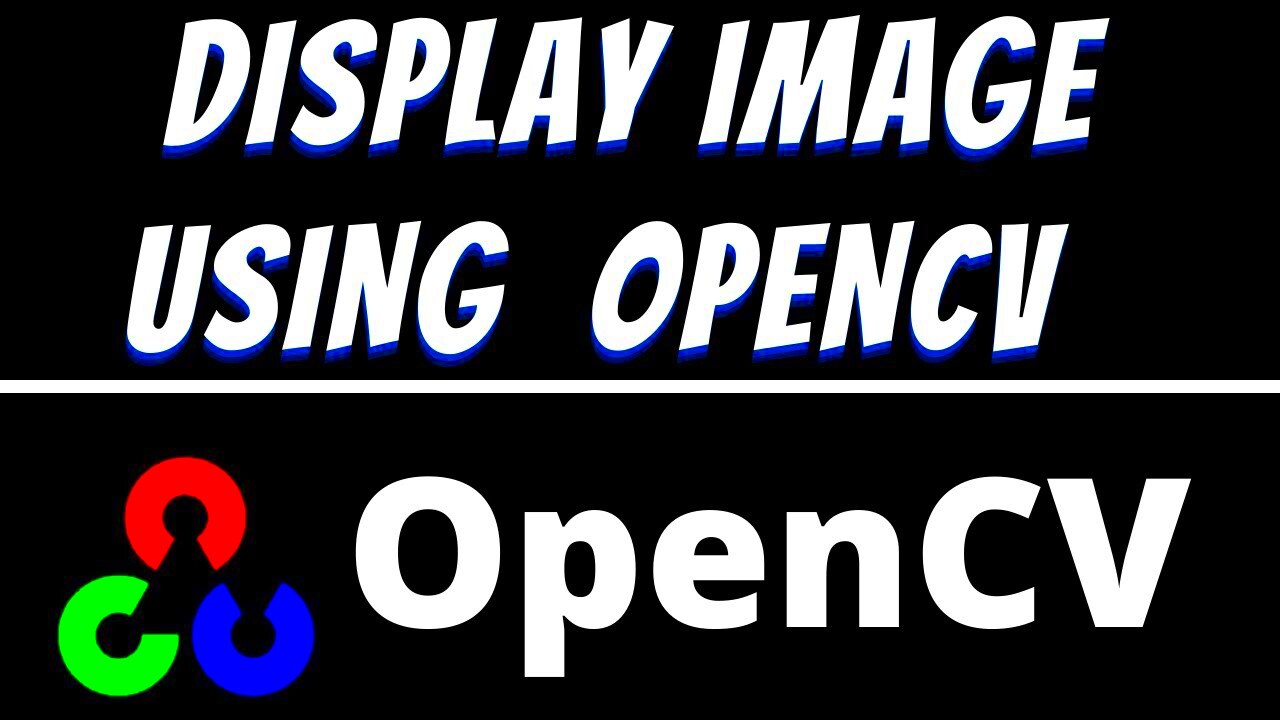
Before we can display images, we need to ensure that our Python environment is ready. Here’s how to get started:
- Install Python: If you haven't done so already, download and install Python from the official website.
- Choose an IDE: You can use any Integrated Development Environment (IDE) like PyCharm, Jupyter Notebook, or VSCode.
- Install Necessary Libraries: For image display, you’ll need a few libraries. Use pip to install them:
pip install opencv-python matplotlib
Once you have your environment set up, you're ready to start working with images in Python. Let’s move on to using libraries for image handling.
Using Libraries for Image Handling
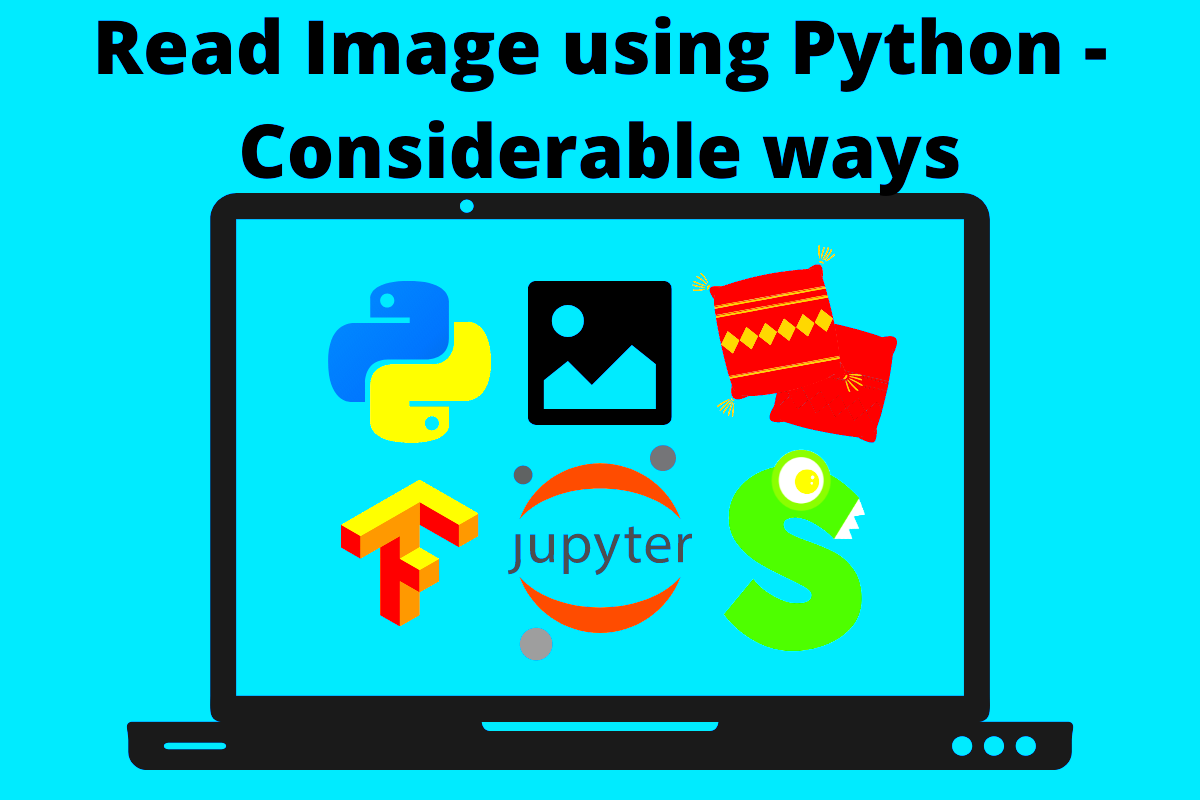
Python offers various libraries for image processing. Two of the most popular ones are OpenCV and Matplotlib. Here’s a quick overview:
Library | Purpose |
---|---|
OpenCV | Great for real-time image processing and computer vision tasks. |
Matplotlib | Perfect for plotting and visualizing data, including images. |
Each library has its strengths. OpenCV is widely used in projects that require complex image analysis, while Matplotlib is ideal for simple image display and data visualization. Depending on your project needs, you may choose one or both libraries to work with images in Python.
Loading Images with OpenCV
OpenCV is a powerful library that makes it easy to work with images and videos in Python. Loading an image with OpenCV is straightforward and requires just a few lines of code. Here's how you can do it:
- Import the OpenCV library: First, ensure you have imported the library in your script.
- Load the image: Use the
cv2.imread()
function to load your image from a file. Specify the path to your image as a string. - Check if the image is loaded: It’s a good practice to check if the image loaded correctly. You can do this by verifying that the image is not
None
.
Here’s a simple example:
import cv2
image = cv2.imread('path_to_your_image.jpg')
if image is not None:
print('Image loaded successfully')
else:
print('Error loading image')
OpenCV supports various image formats, including JPEG, PNG, and BMP. Once you’ve loaded your image, you can proceed to display it or manipulate it further. Let’s see how to display images using Matplotlib.
Displaying Images with Matplotlib
Matplotlib is fantastic for visualizing data, and it’s also very effective for displaying images. It allows you to show images in a way that’s easy to work with. Here’s how to display an image using Matplotlib:
- Import Matplotlib: Use the
import matplotlib.pyplot as plt
command to bring in the library. - Display the image: Use
plt.imshow()
to display the image. Don’t forget to convert the color from BGR to RGB, as OpenCV uses BGR format. - Show the plot: Finally, use
plt.show()
to render the image window.
Here’s a code snippet:
import matplotlib.pyplot as plt
image_rgb = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
plt.imshow(image_rgb)
plt.axis('off') # Hide axis
plt.show()
This code will open a window displaying your image without any axis markings, giving it a cleaner look.
Handling Different Image Formats
When working with images, you’ll encounter various formats. Each format has its characteristics and uses. Here’s a breakdown of some common image formats:
Format | Description | Common Uses |
---|---|---|
JPEG | Compressed format that reduces file size. | Photographs, web images. |
PNG | Supports transparency and lossless compression. | Graphics, logos, and images needing transparency. |
BMP | Uncompressed format that maintains image quality. | Simple graphics, internal processing. |
GIF | Supports animations and limited colors. | Simple animations and graphics. |
OpenCV and Matplotlib can handle most of these formats with ease. Just ensure that you use the correct file extension when loading or saving images. Knowing how to work with different formats allows you to choose the best option for your project, improving efficiency and quality.
Common Issues and Troubleshooting
Working with images in Python can sometimes lead to frustrating issues. Don't worry; these problems are usually easy to fix with a little troubleshooting. Here are some common issues you might encounter:
- Image Not Loading: If your image doesn’t load, check the file path. Make sure it’s correct and that the file exists in that location.
- Image Displayed in Wrong Colors: If your image appears with incorrect colors, remember that OpenCV loads images in BGR format, while Matplotlib uses RGB. Convert your image using
cv2.cvtColor()
. - File Format Errors: Ensure that the file extension matches the actual format of the image. For example, if you have a JPEG image, it should end in
.jpg
or.jpeg
. - Environment Issues: If you encounter library errors, double-check that you have installed the necessary packages using pip.
By being aware of these common problems, you can save time and frustration. Remember, troubleshooting is part of the learning process, so don't hesitate to look for solutions online or consult the documentation.
FAQ
Here are some frequently asked questions about loading and displaying images in Python:
- What is the best library for image processing in Python?
OpenCV is excellent for complex tasks, while Matplotlib is great for basic display and plotting.
- Can I display images in a Jupyter Notebook?
Yes, you can use Matplotlib to display images directly in Jupyter Notebooks.
- What if my image doesn’t show up?
Check your file path, ensure the file format is correct, and confirm that you are using the right library functions.
- Can I save the displayed image?
Yes, you can use
cv2.imwrite()
in OpenCV orplt.imsave()
in Matplotlib to save images.
If you have other questions, consider checking community forums or the official documentation for more detailed information.
Conclusion
Displaying and handling images in Python opens up many possibilities for creativity and analysis. By using libraries like OpenCV and Matplotlib, you can easily load, display, and manipulate images to fit your needs. Remember to troubleshoot common issues as they arise and explore the various image formats available. With practice, you’ll become more comfortable with these tools and enhance your projects significantly. Keep experimenting and have fun with your image processing journey!